SwiftUI offers a variety of semantic colors that adapt to different contexts and system settings,…
View, ViewBuilder and ViewModifier in SwiftUI
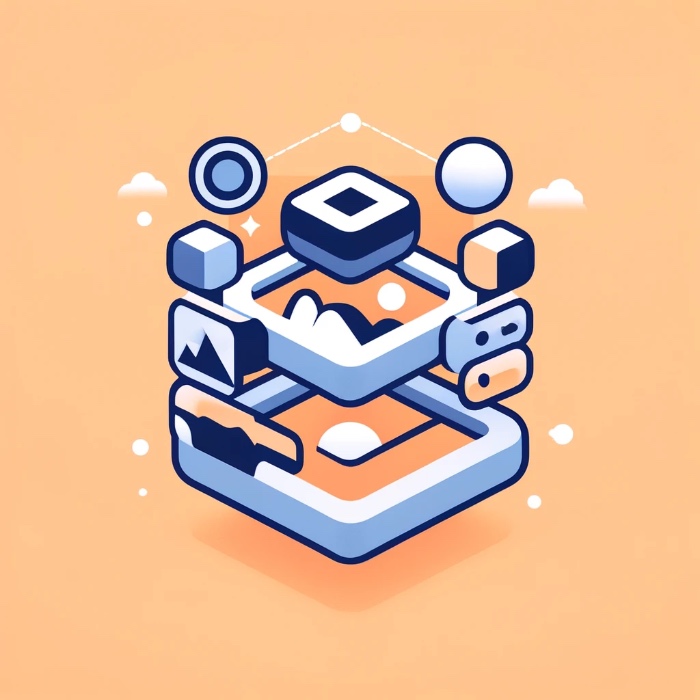
In this tutorial, we will delve into the basics of SwiftUI views, focusing on View, ViewBuilder, and ViewModifier. These concepts are foundational for anyone looking to create robust and reusable UI components in SwiftUI. Let’s break down each term with definitions and follow up with a unique tutorial on how to use these features effectively in your SwiftUI applications.
Definitions
View
A View
in SwiftUI represents a part of the user interface. It conforms to the View
protocol, and you define its appearance and behavior by implementing the required body
computed property. Views
can be composed of built-in SwiftUI views, other custom views, and view modifiers to configure their presentation.
ViewBuilder
ViewBuilder
is an attribute used on closure parameters that produce views, allowing these closures to contain multiple statements and conditionally include views. It simplifies the creation of complex view hierarchies and is typically used to define dynamic UIs where the content might change based on runtime conditions.
ViewModifier
A ViewModifier
is used to create reusable modifications that you can apply to views. By adopting the ViewModifier
protocol, you encapsulate styling and layout modifications in a way that they can be easily reused across different parts of your app.
Tutorial: Building a Custom UI Component with SwiftUI
Step 1: Creating a Custom View
First, we’ll define a simple custom view that displays a greeting message. This view will use basic SwiftUI views and demonstrate how easy it is to define your own view.
struct NewView: View {
var body: some View {
Text("UIExamples.com")
.frame(width: 200, height: 200)
.padding()
.foregroundColor(Color.white)
.font(.title)
.lineLimit(0)
.background(Color.blue)
.clipShape(Circle())
.minimumScaleFactor(0.1)
}
}
NewView
showcases basic SwiftUI functionality, including text display inside a circle and basic styling.
Step 2: Utilizing ViewBuilder
Next, let’s create a component that uses ViewBuilder
to allow for dynamic content creation. We’ll build a NewToolbar
component that can accept an arbitrary number of buttons.
struct NewToolbar<Content: View>: View {
let content: Content
@ViewBuilder var body: some View {
HStack {
content
}
.padding(.horizontal)
.background(Color.blue)
.foregroundColor(.white)
.clipShape(RoundedRectangle(cornerRadius: 10))
}
init(@ViewBuilder content: () -> Content) {
self.content = content()
}
}
struct ContentView: View {
var body: some View {
NewToolbar {
Button("Refresh") { print("Refresh tapped") }
Button("Add") { print("Add tapped") }
}
}
}
Step 3: Creating a Custom ViewModifier
Finally, let’s create a custom ViewModifier
that provides a consistent style for captions across the app.
struct CaptionStyle: ViewModifier {
func body(content: Content) -> some View {
content
.font(.caption)
.padding(10)
.background(.tertiary)
.clipShape(RoundedRectangle(cornerRadius: 8))
}
}
extension View {
func captionStyle() -> some View {
modifier(CaptionStyle())
}
}
struct ContentViewNew: View {
var body: some View {
Text("SwiftUI is awesome!")
.captionStyle()
}
}
This CaptionStyle
modifier can be applied to any view, making it versatile and reusable across your SwiftUI application.
Conclusion
Understanding and utilizing View
, ViewBuilder
, and ViewModifier
are essential for crafting efficient and maintainable SwiftUI applications. By learning these concepts, you enhance your ability to create sophisticated user interfaces with reusable components, significantly boosting your development workflow in SwiftUI.