SwiftUI offers a variety of semantic colors that adapt to different contexts and system settings,…
Toolbar, ToolbarItem, ToolbarItemGroup, and Menu in SwiftUI
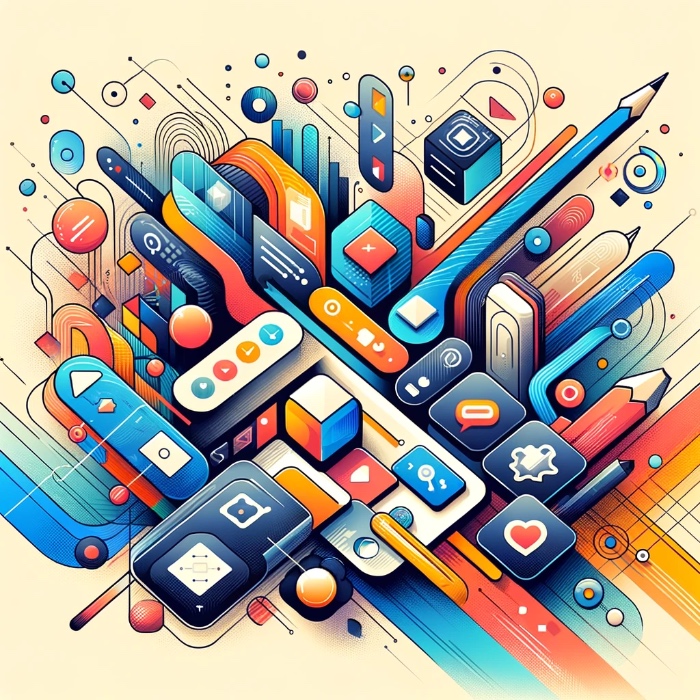
SwiftUI provides a powerful and intuitive way to add and customize toolbars in your app’s user interface.
Toolbar in SwiftUI
Let’s create a simple app interface in SwiftUI that allows users to change the color and font size
struct ContentView: View {
@State private var selectedColor: Color = .black
@State private var fontSize: CGFloat = 15.0
var body: some View {
NavigationStack {
ZStack {
// Placeholder for drawing canvas
Color.white
VStack {
Spacer()
Text("UIExamples.com")
.foregroundColor(selectedColor)
.font(.system(size: fontSize))
Spacer()
}
}
.toolbar {
ToolbarItemGroup(placement: .bottomBar) {
ColorPicker("Font Color", selection: $selectedColor, supportsOpacity: false)
.padding()
Slider(value: $fontSize, in: 10...20) {
Text("Font Size")
}
.frame(width: 150)
}
}
}
}
}
ToolbarItem
ToolbarItem
is used to place individual items or actions within a toolbar. You can specify the placement of these items and customize their content.
Example: Adding a Button to the Toolbar
struct ToolbarItemExample: View {
@State private var text: String?
var body: some View {
NavigationStack {
Text(text ?? "")
.navigationTitle("ToolbarItem Example")
.toolbar {
ToolbarItem(placement: .navigationBarTrailing) {
Button(action: {
print("Action button tapped!")
text = "UIExamples.com"
}) {
Image(systemName: "plus")
}
}
}
}
}
}
In this example, a ToolbarItem
is added to the navigation bar’s trailing edge, represented by a plus icon.
Grouping Multiple Toolbar Items with ToolbarItemGroup
struct SwiftUIToolbarContentExample: View {
@State private var text: String?
var body: some View {
NavigationStack {
Text(text ?? "")
.navigationTitle("ToolbarContent Example")
.toolbar {
ToolbarItemGroup(placement: .bottomBar) {
Button("First") { print("First button tapped")
text = "UIExamples.com: First button tapped"
}
Spacer()
Button("Second") { print("Second button tapped")
text = "UIExamples.com: Second button tapped"
}
}
}
}
}
}
ToolbarItemPlacement and ToolbarTitleMenu
ToolbarItemPlacement
defines where a toolbar item should be located. ToolbarTitleMenu
is not a direct SwiftUI component but can be achieved by using a ToolbarItem
with specific placement to create a menu in the toolbar title’s vicinity.
Example: Creating a Menu in the Toolbar
struct ToolbarMenuExample: View {
@State private var text: String?
var body: some View {
NavigationStack {
Text(text ?? "")
.navigationTitle("Title")
.toolbar {
ToolbarItem(placement: .navigationBarLeading) {
Menu("Options") {
Button("Option 1", action: { print("Option 1 selected")
text = "UIExamples.com: First button tapped"
})
Button("Option 2", action: { print("Option 2 selected")
text = "UIExamples.com: Second button tapped"
})
}
}
}
}
}
}
In this example, a Menu
is placed in the navigation bar’s leading edge using a ToolbarItem
. This creates a dropdown menu with options, mimicking the functionality of a title menu.