SwiftUI offers a variety of semantic colors that adapt to different contexts and system settings,…
TextEditor in SwiftUI
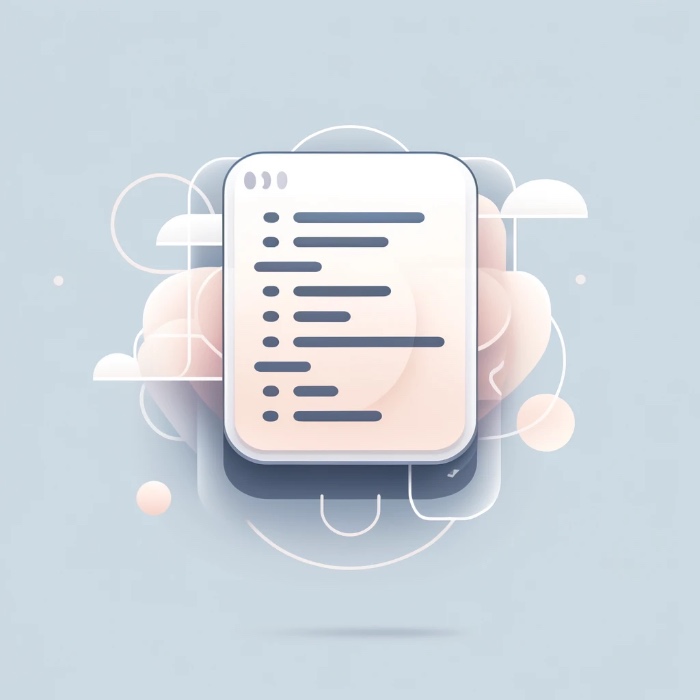
In this tutorial, we’ll explore how to use the TextEditor
in SwiftUI to create a simple yet functional text editing interface. TextEditor
is a versatile component that allows for displaying and editing multiline, scrollable text, making it an essential tool for any app that handles text input. Let’s dive into creating a basic note-taking app where users can input, edit, and style their notes.
Step 1: Setting Up the Text Editor
First, we need to create a SwiftUI view that houses our text editor. We’ll use @State
to bind text data to the TextEditor
, allowing it to be dynamic and editable.
import SwiftUI
struct NotesView: View {
@State private var noteText: String = "Type your notes here..."
var body: some View {
TextEditor(text: $noteText)
.padding()
.border(Color.gray, width: 1)
}
}
In this NotesView
, the TextEditor
is initialized with a binding to the noteText
state variable. This setup allows the text within the TextEditor
to be editable, and any changes made by the user will update the noteText
variable.
Step 2: Styling the Text Editor
Styling the text inside the TextEditor
can enhance the user experience by making the text more readable and visually appealing. You can apply standard SwiftUI modifiers to change the font, color, and line spacing.
struct NotesView: View {
@State private var noteText: String = "Type your notes here..."
var body: some View {
TextEditor(text: $noteText)
.foregroundColor(Color.blue) // Sets the text color to blue
.font(.system(size: 18, weight: .medium, design: .rounded)) // Uses a rounded, medium-weight system font
.lineSpacing(10) // Sets the line spacing to 10 points
.padding()
.border(Color.gray, width: 1)
}
}
Step 3: Adding Functional Modifiers
Sometimes you might want to limit the number of lines the TextEditor
can display or adjust the scaling of the font to fit different screen sizes. For this, SwiftUI provides modifiers like lineLimit
and minimumScaleFactor
.
struct NotesView: View {
@State private var noteText: String = "Type your notes here..."
var body: some View {
TextEditor(text: $noteText)
.foregroundColor(Color.blue)
.font(.system(size: 18, weight: .medium, design: .rounded))
.lineSpacing(10)
.lineLimit(4) // Limits the number of visible lines
.minimumScaleFactor(0.5) // Allows the font size to scale down to 50% if needed
.padding()
.border(Color.gray, width: 1)
}
}
These modifiers ensure that the text editor remains functional and looks good regardless of the amount of text or the device it’s being viewed on.
Conclusion
The TextEditor
in SwiftUI is a powerful tool for creating text input interfaces. By utilizing its flexibility and combining it with other SwiftUI modifiers, you can easily integrate text editing functionalities into your apps. This tutorial demonstrated how to set up, style, and modify a TextEditor
to create a basic yet effective note-taking application. Experiment with different modifiers and settings to tailor the TextEditor
to your app’s needs.