SwiftUI offers a variety of semantic colors that adapt to different contexts and system settings,…
Text Alignment and Layout in SwiftUI
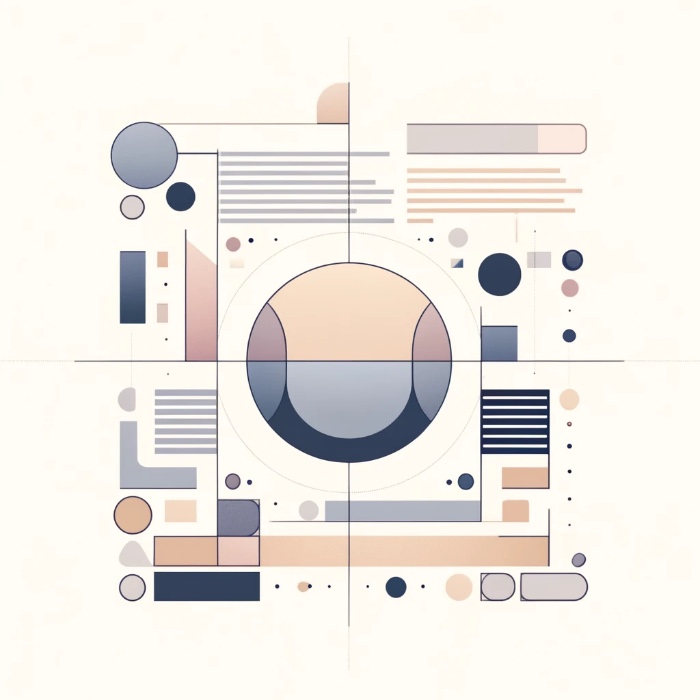
When designing user interfaces with SwiftUI, managing text alignment and layout is crucial for readability and visual appeal. This post will explore effective strategies for text alignment and layout, ensuring your SwiftUI applications are both aesthetically pleasing and functional.
Text Alignment in SwiftUI
SwiftUI provides several alignment options, including leading, trailing, and center. The .multilineTextAlignment(_:)
modifier allows you to specify how text is aligned horizontally within a Text
view.
Here’s an example demonstrating different alignment options for multiline text:
struct TextView: View {
var body: some View {
VStack {
Text("Left \nalignement")
.multilineTextAlignment(.leading)
Text("Right \nalignement")
.multilineTextAlignment(.trailing)
Text("Center \nalignement")
.multilineTextAlignment(.center)
}
.background(.green)
}
}
This setup ensures that text within each Text
view aligns according to the specified alignment option, useful for creating visually balanced layouts.
Line Limit and Truncation
Control the display of long text strings using the .lineLimit(_:)
modifier, which sets the maximum number of lines the text can span. When space is limited, you can combine it with text truncation settings:
struct TruncationExampleView: View {
var body: some View {
Text("This is an example of a long piece of text that might need truncation.")
.lineLimit(2)
.truncationMode(.tail)
.frame(width: 200)
}
}
This text will be truncated at the end if it exceeds two lines, helping maintain a clean and uncluttered UI.
TruncationMode options are: .tail .head and .middle
Layout Considerations
Using stacks and frames, you can precisely control the placement and size of text elements. Combine Text
views with other components for complex layouts.
struct TextLayoutView: View {
var body: some View {
VStack(alignment: .trailing) {
Text("Welcome to the app")
Text("Get started")
}
}
}
Here, the text aligns to the leading edge within a defined frame width, creating a neat and orderly appearance.
Combining Text with Other Views
For more dynamic layouts, combine text views with images, icons, or interactive components using stacks.
struct UserProfileView: View {
var body: some View {
HStack {
Image(systemName: "person.crop.circle.fill")
.font(.largeTitle)
VStack(alignment: .leading) {
Text("Username")
.font(.headline)
Text("Status: Online")
.font(.subheadline)
}
}
}
}
This configuration aligns an icon next to user information, enhancing both functionality and aesthetic appeal.
Accessibility and Dynamic Type
Consider accessibility options like Dynamic Type to adapt font sizes based on user settings, ensuring accessibility for users with varying visual needs.
struct AccessibleTextView: View {
var body: some View {
Text("Adjustable font size text. Some text. Some Text. Some Text. Some Text. Some Text. Some Text. Some Text. Some Text. Some Text.")
.font(.body)
.fixedSize(horizontal: false, vertical: true)
.frame(width: 200, height: 100)
.border(Color.red)
.padding(.top))
}
}
This approach respects user font size preferences, making your app more accessible and user-friendly.
Conclusion
Effective text alignment and layout are vital for crafting polished and accessible interfaces in SwiftUI. By utilizing SwiftUI’s comprehensive set of layout modifiers and considering user accessibility needs, you can create applications that are not only visually engaging but also inclusive. Explore various alignment and layout strategies to find the best fit for your app’s design goals.