SwiftUI offers a variety of semantic colors that adapt to different contexts and system settings,…
SwiftUI ZStack Layering Techniques
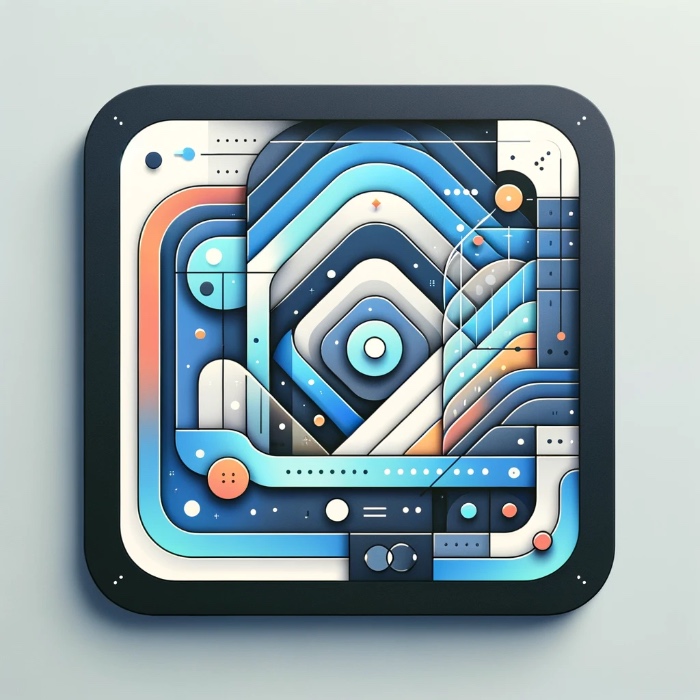
ZStack
is a SwiftUI container that layers its child views along the z-axis. It’s useful for creating overlays, backgrounds, and complex layered interfaces. Views in a ZStack
are stacked in the order they are declared, with the first view being at the bottom and the last one at the top.
Setting Backgrounds in SwiftUI
Before diving into ZStack
, let’s understand how to set backgrounds for views in SwiftUI. You can use the .background()
modifier to set a view’s background. This can be a color, an image, or even another view.
Example: Setting a Background
Text("Hello, SwiftUI!")
.font(.largeTitle)
.background(Color.blue)
Here, the text “Hello, SwiftUI!” will have a blue background.
Using ZStack for Layering Views
Now, let’s explore how to use ZStack
for creating layered interfaces.
Example: Simple ZStack
ZStack {
Color.green
Text("On top of Green")
}
In this example, the text is layered on top of a green background.
Example: Complex Layering
ZStack {
Image("backgroundImage")
VStack {
Text("Title")
Text("Subtitle")
}
.padding()
.background()
.cornerRadius(10)
}
Here, an image is used as the background with text layered over it. You need to have backgroundImage in your Assests in Xcode.
Best Practices for Using ZStack
- Order Matters: Remember that the first view you add to the
ZStack
will be at the bottom, and the last one will be at the top. - Use Opacity Wisely: If you want underlying layers to be visible, adjust the opacity of the views on top.
- Alignment: By default,
ZStack
centers its content. You can change this using the alignment parameter. - Performance: Be mindful of performance. Overusing
ZStack
with complex views can impact your app’s performance. - Accessibility: Consider accessibility. Ensure that text and important elements are not obscured by other layers.
Conclusion
ZStack
in SwiftUI opens up a world of possibilities for creative UI design. By understanding how to layer views and set backgrounds, you can create interfaces that are both functional and aesthetically pleasing. Experiment with different combinations to discover what works best for your app.