SwiftUI offers a variety of semantic colors that adapt to different contexts and system settings,…
SwiftUI vs UIKit – Declarative vs. Imperative Programming
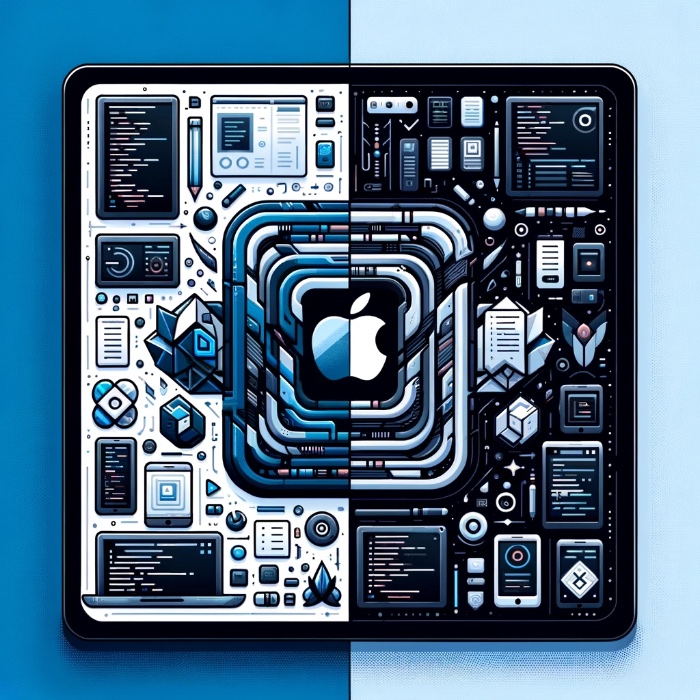
In the world of iOS development, the introduction of SwiftUI brought a significant paradigm shift. SwiftUI, with its declarative nature, presents a contrasting approach to the older, imperative style of UIKit. Understanding the differences between these two frameworks is crucial for iOS developers. In this blog post, we will explore the main differences between declarative and imperative programming concepts as they apply to SwiftUI and UIKit, and how views are updated in both scenarios.
1. What is Declarative Programming (SwiftUI)?
SwiftUI is Apple’s framework introduced in 2019 for developing user interfaces in a declarative way. In declarative UI, you describe what the UI should look like, not how to create it. You declare your user interface and its behavior in a way that is much closer to natural language, using SwiftUI’s body property.
Key Characteristics of SwiftUI:
- Less Code: SwiftUI requires fewer lines of code to achieve the same result as UIKit.
- Data-driven: The UI components are a function of the state. When the state changes, the UI updates automatically.
- Consistency Across Platforms: SwiftUI works across all Apple platforms, providing a unified development experience.
- Live Preview: With SwiftUI, you can see live previews of your UI as you code.
import SwiftUI
struct ContentView: View {
@State private var isButtonPressed = false
var body: some View {
Button(action: {
self.isButtonPressed.toggle()
}) {
Text(isButtonPressed ? "Pressed!" : "Press Me")
}
}
}
In this SwiftUI code, we describe a Button
and its behavior. The UI automatically updates when isButtonPressed
changes, without any need for explicit UI manipulation code.
2. What is Imperative Programming (UIKit)?
UIKit, the traditional framework used in iOS development, is imperative. This means you instruct the program how to perform operations to achieve the desired UI. You manually update the UI based on the state of the application, often leading to more boilerplate code.
Key Characteristics of UIKit:
- Detailed Control: UIKit gives you granular control over UI elements and their behaviors.
- Familiarity and Maturity: Being older, UIKit has a vast community, resources, and a proven track record.
- Manual Updates: Changes in the UI need to be manually handled, requiring more lines of code and effort.
import UIKit
class ViewController: UIViewController {
private var isButtonPressed = false
private let button = UIButton()
override func viewDidLoad() {
super.viewDidLoad()
setupButton()
}
private func setupButton() {
button.setTitle("Press Me", for: .normal)
button.addTarget(self, action: #selector(buttonTapped), for: .touchUpInside)
// additional code to layout the button
}
@objc private func buttonTapped() {
isButtonPressed.toggle()
updateButtonTitle()
}
private func updateButtonTitle() {
button.setTitle(isButtonPressed ? "Pressed!" : "Press Me", for: .normal)
}
}
In the UIKit example, we manually manage the state and update the UI (button title) based on the user interaction.
3. SwiftUI vs UIKit – Main Differences
Declarative vs. Imperative:
- SwiftUI: You declare the UI elements and their dependencies on data (datasource). The framework takes care of the rest.
- UIKit: You explicitly create and manage UI elements and their lifecycle.
State Management and UI Updates:
- SwiftUI: The view is a function of the state. Any changes in the state lead to a re-render of the UI.
- UIKit: You have to manually listen to changes and update the UI accordingly.
Code Conciseness and Readability:
- SwiftUI: Less and more readable code. Easier for beginners and reduces the chance of bugs.
- UIKit: More verbose, offering finer control but at the cost of more code.
4. How Views Update in SwiftUI and UIKit
SwiftUI:
In SwiftUI, the view automatically updates when the state changes. SwiftUI’s view is a struct, which is a value type, ensuring a single source of truth. When the underlying data changes, SwiftUI intelligently re-renders the view to reflect those changes, making the data flow seamless and straightforward.
UIKit:
In UIKit, updating the view is a manual process. When the data changes, you need to write additional code to update the UI elements. This approach gives you more control but requires a deeper understanding of the view lifecycle and state management.
Conclusion
In summary, SwiftUI’s declarative nature offers a modern, efficient, and streamlined approach to UI development in iOS, ideal for rapid development and reducing boilerplate code. UIKit, with its imperative approach, provides detailed control and is backed by years of community support and resources. Both have their place in iOS development, and the choice largely depends on the project requirements and developer preference.