SwiftUI offers a variety of semantic colors that adapt to different contexts and system settings,…
SwiftUI Navigation Patterns for Modern App Architecture
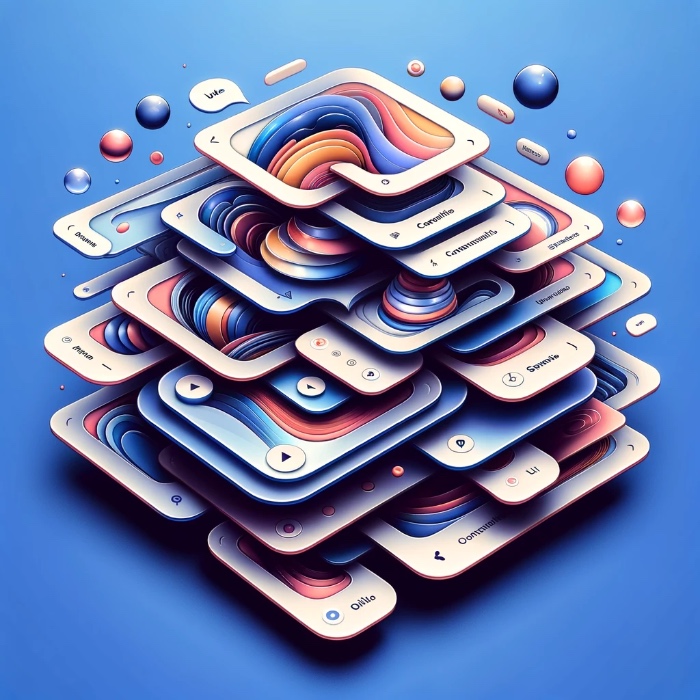
In the evolving landscape of iOS development, mastering SwiftUI navigation patterns is crucial for crafting apps that stand out in both functionality and design. Let’s dive into the core of building seamless navigation within your apps, tailored for the modern user experience. As we unravel the mysteries of SwiftUI’s navigation capabilities, this guide aims to serve as your compass, navigating through the various patterns that shape the backbone of any well-architected application.
Stack Navigation with NavigationStack and NavigationLink
Stack navigation is foundational in SwiftUI, allowing users to navigate through hierarchical content structures.
import SwiftUI
struct ContentView: View {
var body: some View {
NavigationStack {
List {
NavigationLink("Detail View", destination: DetailView())
}
.navigationTitle("Stack Navigation")
}
}
}
struct DetailView: View {
var body: some View {
Text("This is the Detail View")
}
}
To learn more about using NavigationLink read this guide
Tab Navigation with TabView
Tab navigation is ideal for apps with several parallel sections of content or functionality.
import SwiftUI
struct ContentView: View {
var body: some View {
TabView {
Text("Home")
.tabItem {
Label("Home", systemImage: "house")
}
Text("Settings")
.tabItem {
Label("Settings", systemImage: "gear")
}
}
}
}
Modal Presentations with .sheet
Modal presentations are used for tasks that require completion before returning to the main flow.
import SwiftUI
struct ContentView: View {
@State private var showingSheet = false
var body: some View {
Button("Show Modal") {
showingSheet.toggle()
}
.sheet(isPresented: $showingSheet) {
ModalView()
}
}
}
struct ModalView: View {
var body: some View {
Text("Modal Content")
}
}
Master-Detail Interface with NavigationSplitView
The master-detail interface is great for apps that need to display a list of items alongside their detailed content, especially on larger screens.
import SwiftUI
// Detail view displaying a simple message
struct DetailView: View {
let message: String
var body: some View {
Text(message)
.padding()
}
}
// Main ContentView with NavigationSplitView
struct ContentView: View {
@State private var selectedOption: String?
var body: some View {
NavigationSplitView {
List {
Button("Option 1") {
selectedOption = "Detail for Option 1"
}
Button("Option 2") {
selectedOption = "Detail for Option 2"
}
}
} detail: {
if let selectedOption = selectedOption {
DetailView(message: selectedOption)
} else {
Text("Select an option")
}
}
}
}
Page-Based Navigation with TabView and PageTabViewStyle
Page-based navigation is suited for sequential content, like tutorials or galleries. To switch style change indexDisplayMode parameter from .always to .never.
struct ContentView: View {
var body: some View {
TabView {
ForEach(0..<5) { num in
Text("Page \(num + 1)")
.frame(maxWidth: .infinity, maxHeight: .infinity)
.foregroundStyle(.white)
.background(.green)
}
}
.ignoresSafeArea()
.tabViewStyle(PageTabViewStyle(indexDisplayMode: .always))
}
}
Conclusion
Each navigation pattern we’ve discussed serves a unique purpose, catering to different app structures and user needs. Whether it’s guiding users through a learning curve with page-based navigation or organizing complex information with master-detail interfaces, the choice of navigation strategy is pivotal in shaping how users interact with your app.
As you venture forward in your SwiftUI endeavors, remember that the art of app development lies not just in the code you write but in the experiences you create. The navigation patterns highlighted here are your building blocks for constructing intuitive, accessible, and enjoyable paths through your apps. Embrace these tools, experiment with their possibilities, and continue to refine your craft. The journey of innovation in app design is endless, and with SwiftUI, you’re well-equipped to navigate its evolving landscape.