SwiftUI offers a variety of semantic colors that adapt to different contexts and system settings,…
SwiftUI Modal Navigation: sheet, fullScreenCover, popover
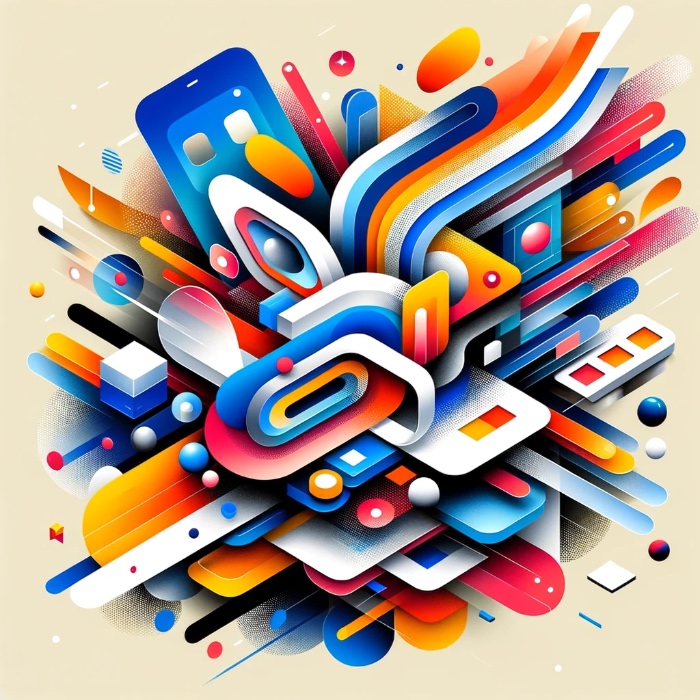
In SwiftUI, modal presentations are a staple for displaying information or gathering input from the user without navigating away from the current view context. Modal presentations can take various forms, including sheets, full-screen covers, and popovers, each suitable for different use cases.
Using isPresented
for Modal Presentations
The isPresented
binding controls the presentation state of a modal. It’s a Boolean value that, when true, presents the modal view and, when false, dismisses it.
Presenting a Modal Sheet
A modal sheet slides up from the bottom of the screen and can be dismissed by the user. It’s ideal for settings, detail information, or input forms.
struct ContentView: View {
@State private var showSheet = false
var body: some View {
Button("Show Sheet") {
showSheet.toggle()
}
.sheet(isPresented: $showSheet) {
// The content of the sheet goes here
Text("Hello, UIExamples.com Friend!")
}
}
}
Using Full-Screen Covers
Full-screen covers take up the entire screen, providing a more immersive experience. They’re perfect for welcome screens or completing tasks without distractions.
struct ContentView: View {
@State private var showFullScreenCover = false
var body: some View {
Button("Show Full-Screen Cover") {
showFullScreenCover.toggle()
}
.fullScreenCover(isPresented: $showFullScreenCover) {
// The content of the full-screen cover goes here
Text("We are Full Screen\n UIExamples.com\n Friend!")
}
}
}
Displaying Popovers
Popovers are small views that appear floating over the current content, typically used on iPad or macOS to present additional information or options.
struct SwiftUIModalNavigation3: View {
@State private var showPopover = false
var body: some View {
Button("Show Popover") {
showPopover.toggle()
}
.popover(isPresented: $showPopover) {
// The content of the popover goes here
Text("More Info Here")
.frame(width: 200, height: 200)
}
}
}
Dismissing Modal Presentations
In SwiftUI, you can programmatically dismiss a modal presentation using the dismiss
action provided by the environment. This is particularly useful in navigation flows or after performing a task.
struct DetailViewForModalNavigation: View {
@Environment(\.dismiss) var dismiss
var body: some View {
Button("Dismiss") {
dismiss()
}
}
}
Understanding presentationDetents(_:)
When you use presentationDetents(_:)
, you define a set of PresentationDetent
values that your sheet supports. This can significantly enhance the usability of sheets within your app, as it allows for multiple resting heights. For example, a sheet might have a .medium
and a .large
detent, enabling the user to choose how much of the sheet is visible based on the content’s relevance or their current focus.
struct PlayerView: View {
@State private var showingLyrics = false
var body: some View {
VStack {
Button("Show Lyrics") {
showingLyrics = true
}
}
.sheet(isPresented: $showingLyrics) {
LyricsView()
.presentationDetents([.medium, .large])
}
}
}
struct LyricsView: View {
var body: some View {
Text("Here are the lyrics...")
}
}
Conclusion
Modal presentations in SwiftUI are powerful tools for enhancing user interaction within your app. By understanding how to use sheets, full-screen covers, and popovers, along with managing their presentation state through isPresented
and programmatically dismissing them with dismiss
, you can create intuitive and engaging interfaces.
Remember, the choice between a sheet, full-screen cover, and popover depends on the amount of content you need to display and the context of use. Experiment with these modal types to find what works best for your app’s user experience.