SwiftUI offers a variety of semantic colors that adapt to different contexts and system settings,…
SwiftUI Label
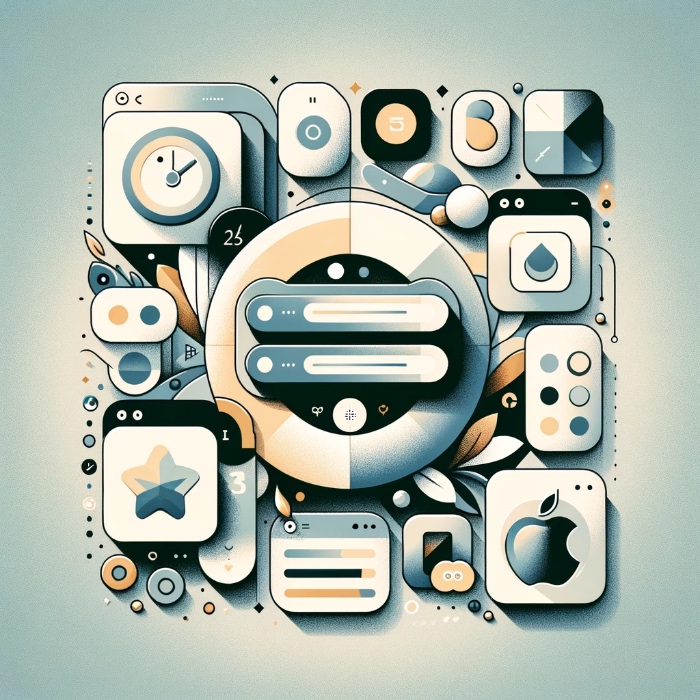
A Label in SwiftUI is a concise way to display an item with an accompanying icon, making information easily digestible and visually appealing. Label View is particularly effective in contexts where space efficiency and user accessibility are priorities, such as in lists, menus, and toolbars.
Why Use Labels?
- Accessibility: Labels are designed with accessibility in mind, supporting features like VoiceOver out of the box.
- Consistency: They help maintain UI consistency across iOS, macOS, watchOS, and tvOS.
- Efficiency: Labels reduce the complexity of combining text and images, simplifying the code and improving readability.
Creating Your First Label
To get started with a Label, you need to specify the text and the icon. Here’s the simplest form of a Label:
Label("Favorites", systemImage: "star.fill")
Customizing Labels
SwiftUI offers flexibility in customizing Labels, allowing you to adjust their appearance and behavior to fit your app’s design language.
Text and Icon Styles
You can modify the text and icon in a Label independently, applying fonts, colors, and other SwiftUI modifiers:
Label {
Text("Alert")
.foregroundColor(.red)
.font(.headline)
} icon: {
Image(systemName: "exclamationmark.circle.fill").foregroundColor(.yellow)
}
This example customizes both the text and icon colors and fonts, showcasing the adaptability of Labels.
Label Styles
SwiftUI provides several built-in label styles for different contexts, such as .iconOnly
and .titleOnly
:
Label("Settings", systemImage: "gear")
.labelStyle(.iconOnly)
This will display only the gear icon, useful for toolbar items or when space is limited.
Advanced: Combining Labels with Navigation
Labels can be seamlessly integrated with navigation elements in SwiftUI, enhancing the user experience by providing clear, actionable items.
NavigationLink with Labels
Use a Label within a NavigationLink
to create intuitive navigational cues:
NavigationLink(destination: DetailView()) {
Label("Go to Details", systemImage: "arrow.right.circle")
}
This setup uses a Label to represent the navigation link visually, combining descriptive text with a relevant icon.
Using Labels with Lists
Labels are particularly useful in List views, where they can serve as row items providing detailed information. Here’s a quick example:
List {
Label("Messages", systemImage: "message.fill")
Label("Settings", systemImage: "gear")
}
Labels in Buttons
Labels are not limited to static displays; they can also be part of interactive elements like buttons. Here’s how you can use a Label within a button to create a visually rich interactive element:
Button(action: {
print("Button was tapped")
}) {
Label("Tap Me", systemImage: "hand.tap.fill")
.foregroundColor(.white)
.padding()
.background(Color.blue)
.cornerRadius(10)
}
Custom Labels with Custom Views
While SwiftUI provides a vast library of system images to use with Labels, you might sometimes need to create a Label with a custom view. Here’s an example:
Label {
Text("Profile")
.font(.title)
.foregroundColor(.green)
} icon: {
RoundedRectangle(cornerRadius: 10)
.fill(Color.green)
.frame(width: 50, height: 50)
.overlay(
Text("P")
.font(.title)
.foregroundColor(.white)
)
}
Labels in Context Menus
Labels can enhance context menus by providing identifiable actions with text and icons. Here’s a simple example:
Text("Long press me")
.contextMenu {
Label("Copy", systemImage: "doc.on.doc.fill")
Label("Delete", systemImage: "trash.fill")
}
Labels in Toolbars
SwiftUI also allows Labels to be used within toolbars for identifiable actions. Here’s an example:
NavigationStack {
Text("Some Text")
.toolbar {
ToolbarItem(placement: .navigationBarTrailing) {
Label("Edit", systemImage: "pencil")
}
}
}
These examples showcase the flexibility of Labels in SwiftUI, demonstrating how they can be integrated into various components and UI designs. By combining text and icons, Labels provide a concise, informative way to enhance the user interface of your apps.