String Interpolation in Swift is a method for constructing new String values by embedding expressions…
Swift Variables and Constants
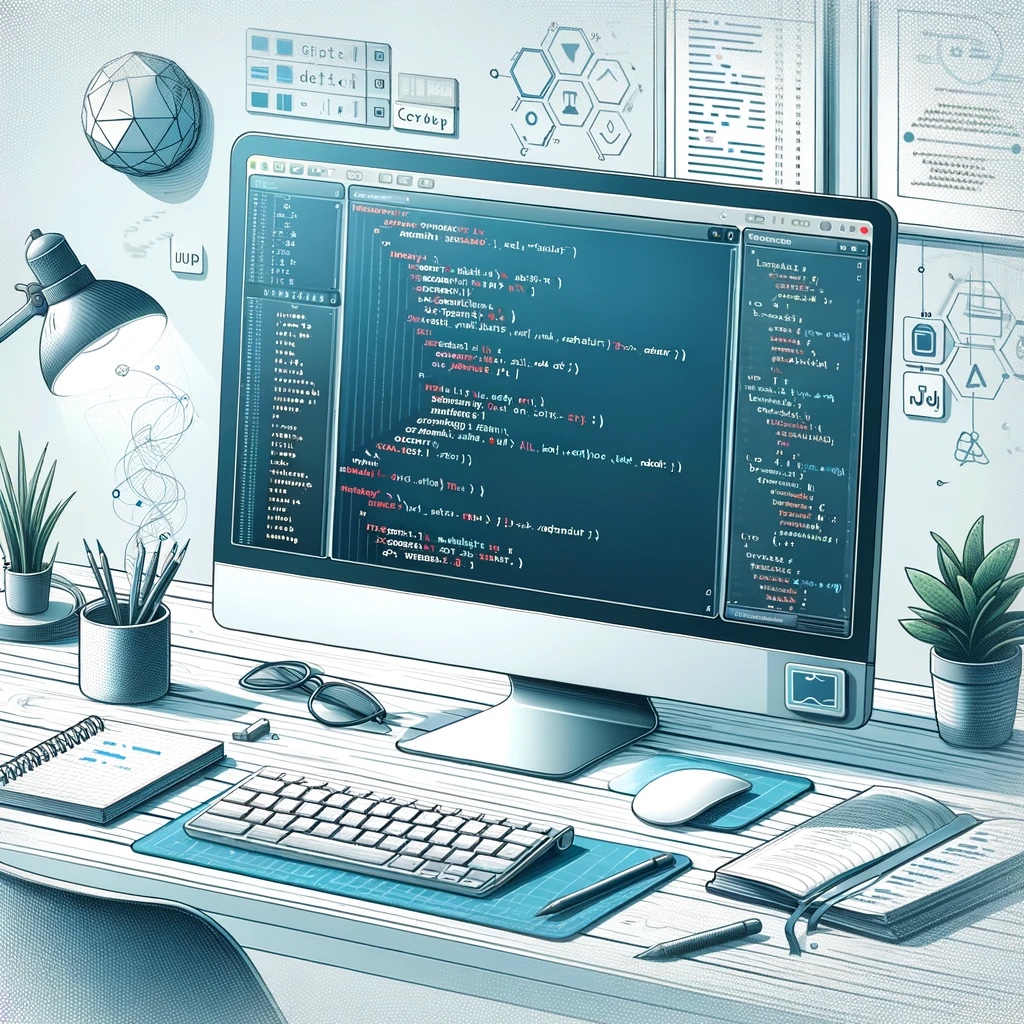
Welcome to UI Examples, your go-to resource for mastering Swift and SwiftUI! Today, we’re delving into one of the fundamental concepts in Swift programming: Constants and Variables. Whether you’re just starting your journey in iOS development or brushing up your skills, understanding these basics is crucial.
What are Variables and Constants?
In Swift, variables and constants are the building blocks of your code. They store information that your program can use and manipulate. But how are they different?
Variables: Your Flexible Friend
Variables in Swift are declared using the var
keyword. Think of variables as containers that can hold different values over time. Here’s how you define a variable:
var playerName = "Alex"
Here, playerName
is a variable that currently holds the value "Alex"
. But the beauty of variables is that they can change, like this:
playerName = "Jordan"
Now, the playerName
has a new value: "Jordan"
.
Constants: Unchanging and Reliable
Constants, on the other hand, are declared with the let
keyword and are used to store values that never change. Once set, a constant’s value is fixed. Here’s how you create a constant:
let pi = 3.14159
Trying to change the value of pi
later in your code will result in an error because pi
is a constant.
Why Use Constants and Variables?
Understanding when to use constants and variables is key to writing clean, efficient, and error-free code in Swift:
- Use a Variable: When you expect the value to change, like a player’s score in a game.
- Use a Constant: When the value should remain the same throughout the program, like the number of days in a week.
Real-World Example: Planning a Party
Let’s put constants and variables into a real-world context: planning a party.
var guests = 10
let venue = "Rooftop Cafe"
Here, guests
is a variable because the number of guests might change. But venue
is a constant since the party location is fixed.
Conclusion
Understanding constants and variables is the first step towards mastering Swift. They might seem simple, but they’re fundamental to controlling data flow in your code.
Stay tuned for more tutorials and deep dives into Swift and SwiftUI here at UI Examples. Happy coding!