String Interpolation in Swift is a method for constructing new String values by embedding expressions…
Swift Numeric Types – Int, Double, and Float
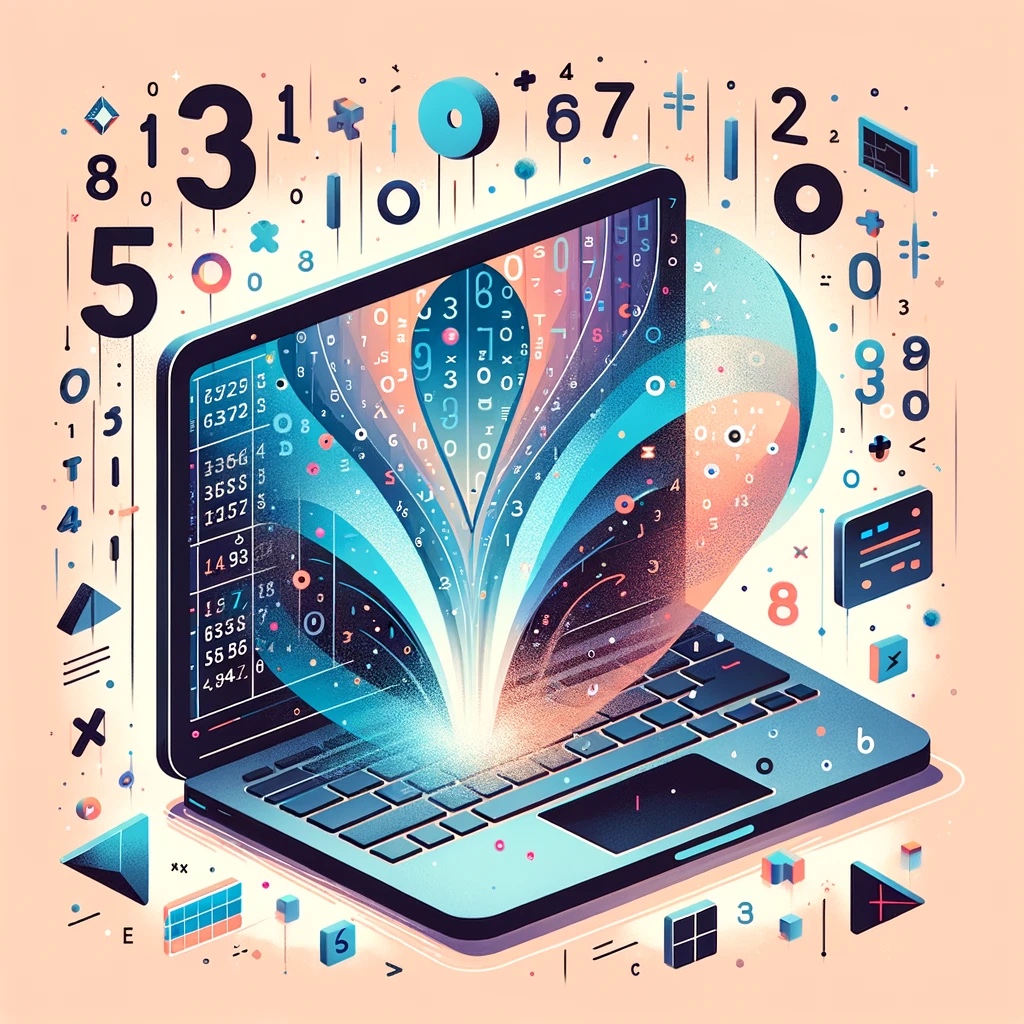
Welcome back to UI Examples, where we unravel the complexities of Swift programming! Today, we’re focusing on the fundamental numeric types in Swift: Int
, Double
, and Float
. Understanding these types is crucial for handling numbers in your Swift code effectively.
Integers (Int
)
What is Int
?
Int
represents whole numbers, which means they do not have a fractional component. In Swift,Int
is used for integers like 3, -99, or 42.
When to Use Int
?
- Use
Int
when you need to count things that can’t be fractional, like the number of people in a room or steps counted by a pedometer.
var attendees = 5
attendees += 2 // attendees now equals 7
var stepsWalked: Int = 3000
stepsWalked += 500
Floating-Point Numbers (Double
and Float
)
What are Double
and Float
?
- Both
Double
andFloat
represent numbers with fractional components (like 3.14 or -23.5). Double
represents a 64-bit floating-point number andFloat
represents a 32-bit floating-point number.
Double
vs. Float
Double
has a higher precision (about 15 decimal digits) thanFloat
(about 6 decimal digits).- Use
Double
for more precise calculations and when dealing with large numbers.
var pi: Double = 3.14159 // Higher precision for mathematical calculations
var opacity: Float = 0.9 // Sufficient for UI element transparency
When to Use Them?
- Use
Double
orFloat
for measurements, like distance, speed, or temperature.
var distance: Double = 24.5 // Distance in miles
var temperature: Float = 36.6 // Body temperature in Celsius
Key Differences
- Precision:
Int
is precise since it deals with whole numbers.Float
andDouble
can have rounding errors due to their nature of representing fractional numbers. - Usage: Choose
Int
for counts,Double
for precise calculations or large numbers, andFloat
where memory efficiency is more critical than precision.
Conclusion with Practical Advice
Understanding Int
, Double
, and Float
in Swift opens up a world of possibilities for handling different numeric operations. Whether you’re counting, measuring, or calculating, using the appropriate type is crucial for accurate and efficient programming. Remember, Int
is for whole numbers, Double
for high precision, and Float
for less demanding calculations. Always choose the type that best fits your use case.
Explore these examples and experiment on your own to get a deeper understanding. Stay tuned for more Swift tutorials here at UI Examples!