String Interpolation in Swift is a method for constructing new String values by embedding expressions…
Swift Classes and Structs
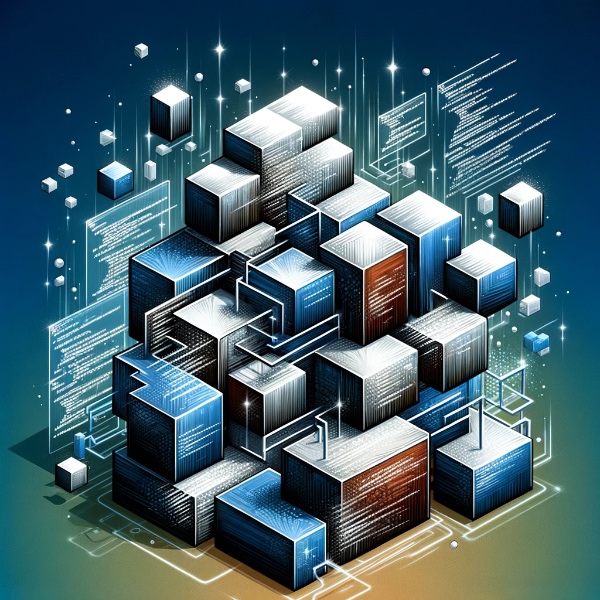
In this post, we’ll explore two fundamental concepts in Swift: Classes and Structs.
What are Swift Classes and Structs ?
In Swift, Classes and Structs are both used to create complex data types, but they have some key differences.
Swift Class
A Swift class is a blueprint for creating objects (known as instances) and defines properties (which are the data) and methods (which are the functions) associated with the type. Classes in Swift have several key characteristics:
- Reference Type: When you create an instance of a class and assign it to a variable or constant, you are actually assigning a reference to that instance. If you assign it to another variable or constant, both refer to the same instance. Changing one will affect the other.
- Inheritance: Classes support inheritance, meaning a class can inherit properties, methods, and other characteristics from another class.
- Deinitialization: Classes have deinitializers, which are called when an instance of the class is about to be destroyed, allowing for cleanup of resources.
- Reference Counting: Swift uses reference counting for class instances to manage memory, keeping track of the number of references to each class instance.
Example of a Swift Class:
class Animal {
var species: String
init(species: String) {
self.species = species
}
func makeSound() {
print("Animal makes a Sound")
}
}
let dog = Animal(species: "Dog")
dog.makeSound()
Swift Struct
A Swift struct is also a blueprint for creating objects, and like classes, structs have properties and methods. However, they differ from classes in some key ways:
- Value Type: Structs are value types, which means when you create an instance of a struct and assign it to a variable or constant, it creates a copy of the data. If you assign this instance to another variable or constant, it copies this data, so changing one does not affect the other.
- No Inheritance: Structs do not support inheritance. They cannot inherit properties, methods, or other characteristics from another struct or class.
- Mutability Control: If an instance of a struct is assigned to a constant, its properties cannot be changed, even if they are declared as variables.
Example of a Swift Struct:
struct Point {
var x: Int
var y: Int
}
var point1 = Point(x: 10, y: 20)
var point2 = point1 // This is a copy of point1
point2.x = 30
print(point1.x) // Outputs "10", point1 remains unchanged
Here, Point
is a struct with two properties. When point1
is copied to point2
, modifying point2
does not change point1
.
struct Point {
var x: Int
var y: Int
}
var point1 = Point(x: 10, y: 20)
var point2 = point1 // This creates a copy of point1
point2.x = 30 // Changing point2 does not affect point1
Key Differences
Example: Shared vs. Unique Instances
With classes (reference types), changing the copy changes the original:
class User {
var name: String
init(name: String) {
self.name = name
}
}
let user1 = User(name: "Alice")
let user2 = user1 // user2 is a reference to the same instance as user1
user2.name = "Bob"
print(user1.name) // Outputs "Bob"
With structs (value types), each copy is independent:
struct Rectangle {
var width: Int
var height: Int
}
var rect1 = Rectangle(width: 100, height: 200)
var rect2 = rect1 // rect2 is a separate copy
rect2.width = 150
print(rect1.width) // Outputs "100"
Conclusion
Understanding the differences between classes and structs in Swift is crucial for effective coding. While classes offer more complexity with inheritance and reference semantics, structs provide simplicity and efficiency with value semantics.
Embrace both in your Swift journey and discover the endless possibilities in app development. Stay tuned to UI Examples for more Swift insights!