String Interpolation in Swift is a method for constructing new String values by embedding expressions…
Swift Array
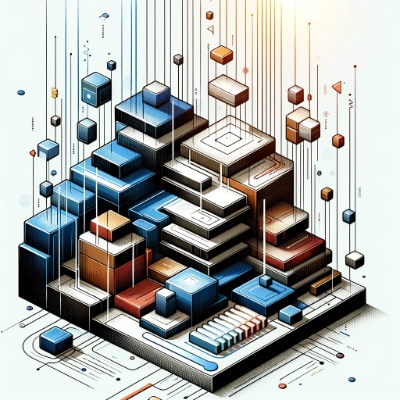
Whether you’re new to Swift or refreshing your skills, this guide will take you through the essentials of Swift array operations with exciting examples.
What is an Array in Swift?
In Swift, an array is a collection of elements that are of the same type. Arrays are ordered, meaning each element has a specific position, often referred to as an index.
Array Operations:
Creating an Array
var fruits = ["Apple", "Banana", "Cherry"]
Appending Elements to an Array
Add new elements to the end.
fruits.append("Dragonfruit")
Inserting Elements to Swift Array
Insert an element at a specific index.
fruits.insert("Blueberry", at: 2)
Removing Elements from Array
Remove an element by specifying its index.
fruits.remove(at: 1) // Removes "Banana"
Counting Elements in Array
Find out how many elements an array has.
let count = fruits.count
print("There are \(count) fruits in the array.")
Accessing Elements
Access elements using their index.
let firstFruit = fruits[0] // "Apple"
let lastFruit = fruits.last // "Dragonfruit"
let firstFruit2 = fruits.first // "Apple"
Iterating Over an Array
Use a for-in
loop to iterate through all elements.
for fruit in fruits {
print("Fruit: \(fruit)")
}
Example: Building a Simple Shopping List App
Let’s create a small example that mimics a shopping list app:
var shoppingList = ["Milk", "Eggs", "Bread"]
// Adding more items
shoppingList.append("Butter")
shoppingList += ["Cheese", "Tomatoes"]
// Iterating over the shopping list
for item in shoppingList {
print("Don't forget to buy \(item)")
}
In this app, we create a shopping list, add more items, and iterate over the list to print what needs to be bought.
Conclusion
Swift arrays are powerful and versatile, perfect for managing ordered collections in your apps. By mastering these basic operations, you can manipulate and utilize arrays effectively in your Swift projects.