SwiftUI offers a variety of semantic colors that adapt to different contexts and system settings,…
Strategy Pattern in SwiftUI
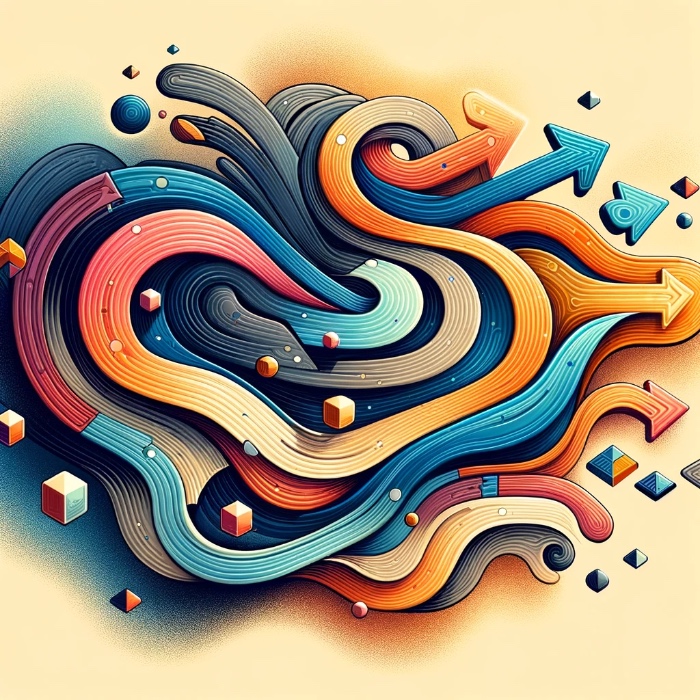
In the rapidly evolving landscape of SwiftUI development, embracing design patterns that offer flexibility and modularity is key to crafting robust and scalable applications. Among these, the Strategy Pattern stands out for its ability to facilitate dynamic behavior changes within your apps, akin to selecting the optimal route for a journey based on current conditions. Let’s dive into how the Strategy Pattern can be ingeniously applied in SwiftUI, enhancing the adaptability and responsiveness of your user interfaces.
Understanding the Strategy Pattern Through SwiftUI
Consider the Strategy Pattern as your navigation app during a road trip, offering various routes (strategies) to your destination. Whether you prefer the scenic route, the fastest one, or the path with the least tolls, the choice is yours, and you can switch preferences seamlessly as you go. In the context of SwiftUI, this pattern enables you to change the behavior of your UI components on the fly, tailoring the user experience to the current context or user preferences without overhauling your app’s structure.
Implementing Text Style Strategies in SwiftUI
To illustrate the practicality of the Strategy Pattern in SwiftUI, we’ll explore an example where users can dynamically switch between different text styles within the app, specifically toggling between bold and italic styles.
Defining the Text Style Strategy Protocol
First, we outline a protocol to serve as a blueprint for our text style strategies, ensuring consistency and interoperability:
protocol TextStyleStrategy {
func applyStyle(to text: String) -> Text
}
Crafting Concrete Strategy Implementations
Next, we develop specific strategies for bold and italic text styles, adhering to the defined protocol:
struct BoldTextStyleStrategy: TextStyleStrategy {
func applyStyle(to text: String) -> Text {
Text(text).fontWeight(.bold)
}
}
struct ItalicTextStyleStrategy: TextStyleStrategy {
func applyStyle(to text: String) -> Text {
Text(text).italic()
}
}
Integrating Strategies into SwiftUI Views
With our strategies in place, we construct a ContentView
that allows users to select their preferred text style through a simple toggle:
struct ContentView: View {
@State private var useBoldText = true
private let text = "Hello, Strategy Pattern!"
var body: some View {
VStack(spacing: 20) {
Text("Choose Text Style:")
Toggle("Use Bold Text", isOn: $useBoldText)
.fixedSize()
if useBoldText {
BoldTextStyleStrategy().applyStyle(to: text)
} else {
ItalicTextStyleStrategy().applyStyle(to: text)
}
}
.padding()
}
}
Learn more: App Design Patterns in SwiftUI