SwiftUI offers a variety of semantic colors that adapt to different contexts and system settings,…
State Pattern, Delegation Pattern, Memento Pattern, Iterator Pattern, Prototype Pattern, Multicast Delegate Pattern, Flyweight Pattern, Mediator Pattern In SwfitUI
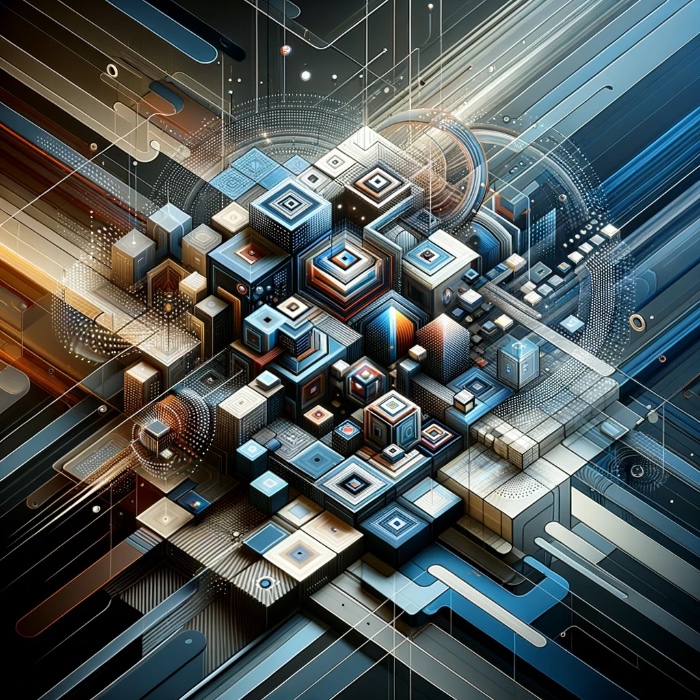
Crafting a detailed guide to lesser-known design patterns with Swift code examples, especially tailored for SwiftUI, presents an exciting opportunity to broaden one’s programming repertoire. Let’s explore these patterns with their descriptions and implement straightforward examples that can be previewed in SwiftUI.
SwiftUI, with its declarative syntax, opens doors to efficiently implementing various design patterns. Let’s delve into some advanced patterns that are not as commonly discussed but can be useful in specific scenarios: State Pattern, Delegation Pattern, Memento Pattern, Iterator Pattern, Prototype Pattern, Multicast Delegate Pattern, Flyweight Pattern, Mediator Pattern.
State Pattern in SwiftUI
Description: The State pattern allows an object to change its behavior when its internal state changes. This pattern is particularly useful in SwiftUI for managing UI components based on the app’s state.
Let’s demonstrate a State Pattern by a simple toggle mechanism controlling the view’s background color.
Step 1: Define the State Protocol and Concrete States
First, we define a basic protocol for our state and then implement two concrete states representing the Active and Inactive states.
protocol BackgroundState {
var backgroundColor: Color { get }
}
struct ActiveState: BackgroundState {
var backgroundColor = Color.green
}
struct InactiveState: BackgroundState {
var backgroundColor = Color.red
}
Step 2: ViewModel to Manage State
Next, we create a ViewModel that will manage the current state and toggle between the Active and Inactive states.
class StateViewModel: ObservableObject {
@Published var currentState: BackgroundState = InactiveState()
func toggleState() {
if currentState is InactiveState {
currentState = ActiveState()
} else {
currentState = InactiveState()
}
}
}
Step 3: SwiftUI View
Finally, we construct a SwiftUI view that uses this ViewModel. A tap gesture on the view toggles the state, and the background color changes accordingly.
struct StatePatternExampleView: View {
@StateObject private var viewModel = StateViewModel()
var body: some View {
VStack {
Text("Tap me to toggle state!")
.foregroundColor(.white)
.padding()
}
.frame(maxWidth: .infinity, maxHeight: .infinity)
.background(viewModel.currentState.backgroundColor)
.onTapGesture {
viewModel.toggleState()
}
}
}
struct StatePatternExampleView_Previews: PreviewProvider {
static var previews: some View {
StatePatternExampleView()
}
}
Simplified Explanation
In this simplified example, the StatePatternExampleView
reacts to tap gestures by toggling its background color between green and red. This behavior is managed by the StateViewModel
, which holds the current state. Tapping the view triggers a state change in the ViewModel, demonstrating the State Pattern’s concept of an object changing its behavior based on its internal state. This approach encapsulates state-specific behaviors, making the SwiftUI view cleaner and focused on UI representation rather than the logic controlling the state transitions.
Delegation Pattern
Description: Delegation allows an object to use another “delegate” object to provide specific functionality. It’s useful in SwiftUI for separating concerns and enhancing reusability.
SwiftUI naturally incorporates delegation through its data flow mechanisms like @Binding
, @State
, and @ObservableObject
. Instead of a direct code example, consider how SwiftUI’s @ObservableObject
and @Published
properties delegate the responsibility of view updates to an observable object.
Memento Pattern
Description: The Memento pattern provides the ability to capture and externalize an object’s internal state so that the object can be restored to this state later. It is useful for implementing undo mechanisms or saving state.
Due to the complexity and less direct relevance to SwiftUI’s common use cases, a simple example would not effectively demonstrate its utility. However, the concept could be applied in managing app states or preferences that need to be restored across app launches.
Iterator Pattern
Description: The Iterator pattern provides a way to access elements of an aggregate object sequentially without exposing its underlying representation. SwiftUI leverages Swift’s for-in
loops and collection protocols, inherently using the Iterator pattern.
Prototype Pattern
Description: This pattern is used for creating new objects by copying existing ones. Swift’s built-in copy
functionality with reference and value types inherently supports this pattern.
Multicast Delegate Pattern
Description: Allows an object to notify multiple delegate objects about state changes. This is akin to SwiftUI’s @Published
property changes being observed by multiple views.
Flyweight Pattern
Description: It minimizes memory usage or computational expenses by sharing as much as possible with related objects; it’s about sharing state. SwiftUI views are inherently designed to be lightweight and reusable, which aligns with the Flyweight pattern’s principles.
Mediator Pattern
Description: The Mediator pattern adds a third party object to control the interaction between two objects, reducing the direct coupling between them. SwiftUI’s environment objects can be seen as mediators, allowing shared data to be accessed by multiple views without tight coupling.
Given the breadth of these patterns and their varying degrees of applicability to SwiftUI, direct, simplified examples for some patterns may not fully convey their utility or may overlap with SwiftUI’s inherent design principles. Each pattern offers unique advantages and can be further explored for specific use cases within app development projects.