SwiftUI offers a variety of semantic colors that adapt to different contexts and system settings,…
State and Binding in SwiftUI
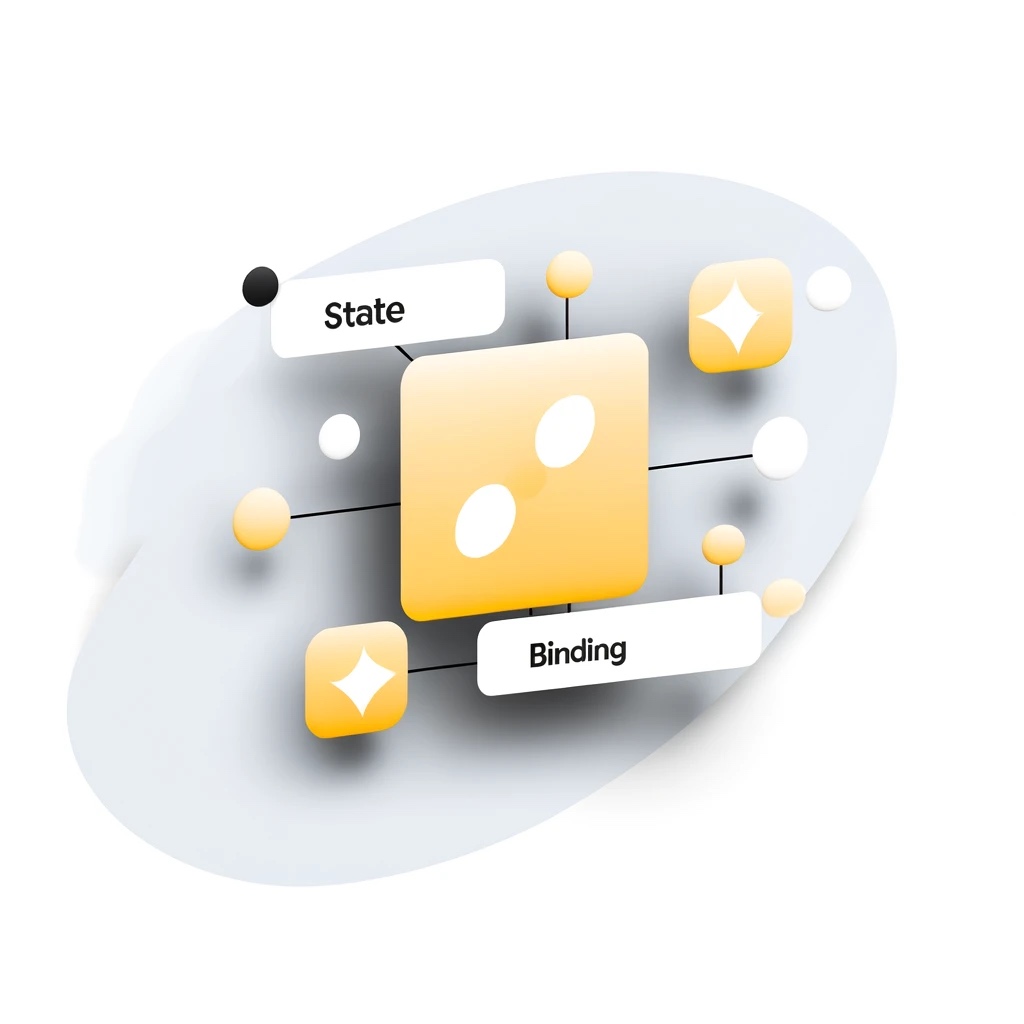
In this tutorial, we’ll delve into how @State
and @Binding
are instrumental in managing data within SwiftUI apps, presenting a practical example that demonstrates their utility in a real-world scenario.
Understanding @State with a Simple Toggle Example
@State
is pivotal for managing local state within a SwiftUI view—it’s the view’s private data storage. Here’s a straightforward example where we use @State
to control the on/off state of a light.
struct LightSwitchView: View {
@State private var isLightOn = false
var body: some View {
VStack {
Text(isLightOn ? "The light is On" : "The light is Off")
Button("Toggle Light") {
isLightOn.toggle()
}
.padding()
.background(isLightOn ? Color.yellow : Color.gray)
.foregroundColor(.white)
.clipShape(Capsule())
}
}
}
In this example, isLightOn
is a @State
variable used to track whether the light is on or off. The button toggles this state, and the view updates to reflect the current state of the light.
Understanding @Binding with a Volume Control Example
@Binding
helps create a two-way connection between a view and data managed elsewhere, making it ideal for cases where data needs to be shared across different views.
Let’s consider a scenario where you have a parent view with a volume control that affects how a child view displays information.
struct SpeakerSystemView: View {
@State private var volume: Double = 50 // Volume level from 0 to 100
var body: some View {
VStack {
Text("System Volume Control")
Slider(value: $volume, in: 0...100)
SpeakerView(volume: $volume)
}
}
}
struct SpeakerView: View {
@Binding var volume: Double
var body: some View {
Text("Speaker volume: \(Int(volume))")
.padding()
.background(volume > 50 ? Color.red : Color.blue)
.foregroundColor(.white)
.clipShape(RoundedRectangle(cornerRadius: 10))
}
}
In the SpeakerSystemView
, volume
is a @State
variable. The SpeakerView
receives this state as a @Binding
, allowing it to reflect and even modify the volume
state directly. Changes in the volume are shared between the parent and child views seamlessly.
Key Differences and When to Use Each
- @State is for internal data management within a view. It is the source of truth within its local context and is not shared directly with other views.
- @Binding is for managing shared data. It does not own the data but instead binds to data owned by another part of your app, allowing multiple views to share and modify the data efficiently.
Conclusion
Understanding and using @State
and @Binding
effectively can greatly enhance the functionality and responsiveness of your SwiftUI apps. They are essential tools for data management, with @State
being perfect for private, view-local data, and @Binding
for shared, interactive data scenarios.
Experiment with the above examples in Xcode’s SwiftUI previews, and try modifying them or creating new ones to deepen your understanding of how @State
and @Binding
work together to manage data flow in SwiftUI applications.