SwiftUI offers a variety of semantic colors that adapt to different contexts and system settings,…
Singleton Pattern in SwiftUI
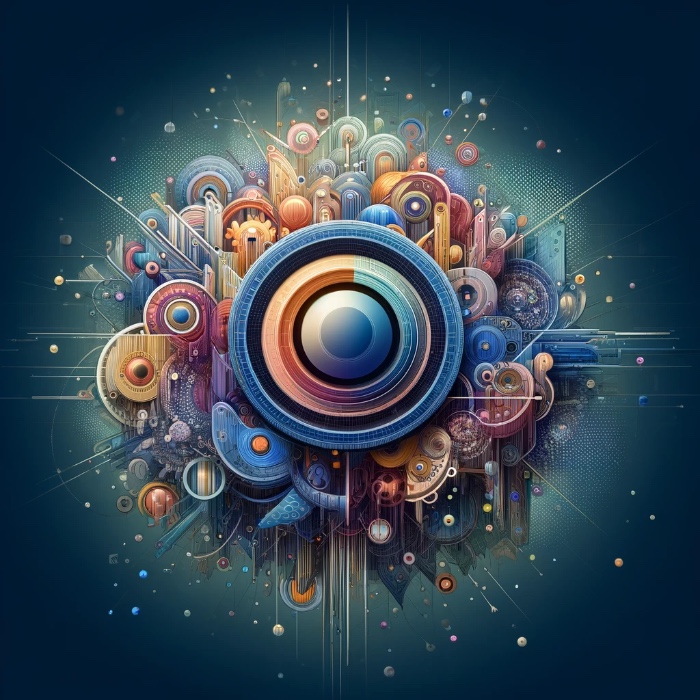
In the world of SwiftUI development, crafting an efficient, coherent, and user-friendly interface is paramount. One approach to achieving such an ideal is through the application of design patterns, among which the Singleton pattern shines for its ability to centralize and manage shared resources effectively. Let’s explore how the Singleton pattern can be seamlessly integrated into a SwiftUI application, enhancing its structure and functionality.
The Singleton Pattern: Your App’s Universal Remote Control
Picture a scenario where you possess a universal remote control capable of commanding every digital device in your space with the push of a button. This remote is the only one of its kind and simplifies the control over your devices, embodying the essence of the Singleton pattern in software development.
This design pattern ensures a class has just one instance while providing a global point of access to that instance. It’s akin to having a single, shared control point for managing app-wide settings or preferences, guaranteeing consistency across your application’s lifecycle.
Implementing the Singleton Pattern in SwiftUI: A User Preferences Case Study
In our case study, we’ll employ the Singleton pattern to manage user preferences within a SwiftUI app. This centralized approach allows for the modification of settings such as notification permissions and background color from anywhere within the app, reflecting changes instantaneously across all views that rely on these preferences.
User Preferences Singleton
Here’s how we define a class to manage user preferences, utilizing the Singleton pattern:
class UserPreferences {
static let shared = UserPreferences()
var notificationsEnabled: Bool = false
var bgColor: Color = .white
private init() {} // Ensures Singleton pattern adherence
}
SwiftUI ContentView
Within the ContentView
, we leverage the Singleton to adapt the app’s settings based on user interactions:
struct ContentView: View {
@State private var notificationsEnabled: Bool = UserPreferences.shared.notificationsEnabled
var body: some View {
ZStack {
UserPreferences.shared.bgColor
Form {
Toggle("Enable Notifications", isOn: $notificationsEnabled)
.onChange(of: notificationsEnabled) { _, newValue in
UserPreferences.shared.notificationsEnabled = newValue
// Toggle the background color between two states
UserPreferences.shared.bgColor = UserPreferences.shared.bgColor == .red ? .blue : .red
}
}
.padding()
}
.ignoresSafeArea()
}
}
In this example, a toggle switch allows users to enable or disable notifications, demonstrating the Singleton pattern’s utility in managing shared application states and preferences. Changes in the toggle state are instantly applied app-wide, thanks to the centralized management of settings through the UserPreferences
class.
Learn more: App Design Patterns in SwiftUI