SwiftUI offers a variety of semantic colors that adapt to different contexts and system settings,…
SensoryFeedback in SwiftUI – Providing Haptic Feedback
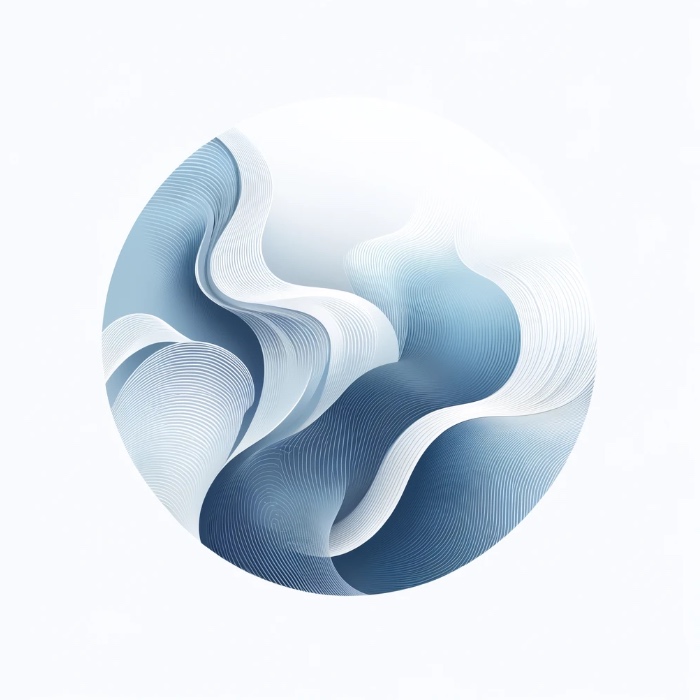
SensoryFeedback
in SwiftUI is a potent feature introduced in iOS 17, enabling developers to provide haptic and audio feedback in response to user interactions. This tutorial will walk through various scenarios to integrate SensoryFeedback
effectively, enhancing the tactile experience of your apps.
Example 1: Success Feedback on Task Completion
When a user completes a task in an app, providing a success feedback can affirm the action positively.
import SwiftUI
struct TaskCompletionView: View {
@State private var isDone = false
var body: some View {
Button("Is Done?") {
isDone = true
}.sensoryFeedback(.success, trigger: isDone)
}
}
This button, when tapped, triggers a success feedback indicating that a task has been successfully completed. This kind of feedback is ideal for to-do lists or goal-tracking apps.
Example 2: Warning Feedback on Potential Issue
struct CriticalValueInputView: View {
@State private var value: Double = 0.0
var body: some View {
VStack {
Slider(value: $value, in: 0...100, step: 1)
.padding()
Text("Current Value: \(value, specifier: "%.0f")")
.padding()
if value > 80 {
Text("Warning: High Value!")
.foregroundColor(.red)
.padding()
.sensoryFeedback(.warning, trigger: value > 80)
}
}
.padding()
}
}
The .sensoryFeedback
modifier in SwiftUI allows developers to incorporate haptic feedback into their apps, providing a tactile response to user interactions. When you use .sensoryFeedback
with the .impact
type, you can specify the weight and intensity of the feedback:
- Impact Weight: Determines how heavy the feedback feels. The weight can be set to different levels such as
.light
,.medium
,.heavy
, etc., each corresponding to the strength of the haptic impact. - Intensity: Adjusts how strong the feedback is. This value is typically a decimal between 0.0 and 1.0, where higher values produce a more forceful feedback.
The trigger
parameter is crucial as it determines when the feedback should occur. This is usually linked to some state in your app, such as a boolean flag or a specific value that changes in response to user actions. When the specified trigger
changes in a way that meets the conditions for feedback (which can be as simple as toggling a boolean or more complex conditions based on user input or app state changes), the haptic feedback is activated
struct FeedbackExampleView: View {
// State variable to trigger feedback
@State private var feedbackTriggered = false
var body: some View {
VStack(spacing: 20) {
Text("Tap the button below to trigger heavy impact feedback.")
.padding()
// Button that toggles the feedbackTriggered state
Button("Trigger Feedback") {
// Toggle the state to trigger feedback
self.feedbackTriggered.toggle()
}
.padding()
.background(Color.blue)
.foregroundColor(.white)
.clipShape(Capsule())
// Attach sensoryFeedback modifier with heavy impact
.sensoryFeedback(.impact(weight: .heavy), trigger: feedbackTriggered)
}
}
}
Conclusion
Integrating SensoryFeedback
in SwiftUI apps not only enriches the user interface but also aids in delivering a more intuitive user experience. By providing immediate, relevant feedback, you can guide users’ actions more effectively and ensure they feel more connected and responsive to the app. Experiment with these examples in your own apps to explore the benefits of enhanced sensory feedback.