SwiftUI's .focused modifier allows you to control and observe the focus state of your views.…
Semantic Colors in SwiftUI: accentColor, primary, secondary, custom color
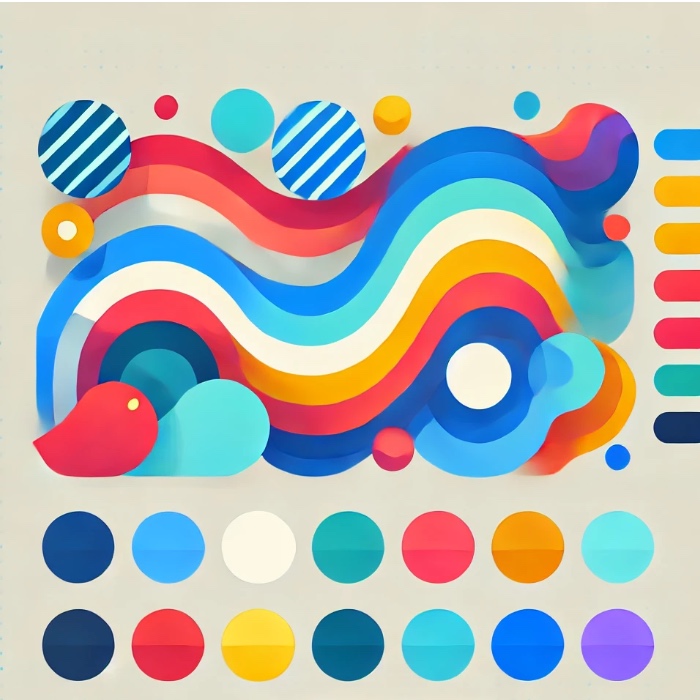
SwiftUI offers a variety of semantic colors that adapt to different contexts and system settings, enhancing the visual consistency and accessibility of your app. Semantic colors automatically adjust to the system’s color scheme and user preferences, making your app look great in both light and dark modes. This tutorial will guide you through using semantic colors in SwiftUI with unique and engaging examples.
Introduction to Semantic Colors
Semantic colors are predefined colors in SwiftUI that adapt to the app’s theme and accessibility settings. Some common semantic colors include accentColor
, primary
, and secondary
.
Example: Using AccentColor
The accentColor
is a broad theme color applied to views and controls. You can set it at the application level by specifying an accent color in your app’s asset catalog.
Setting AccentColor in Asset Catalog
- Open your asset catalog (Assets.xcassets).
- Create a new color set and name it
AccentColor
. - Choose your desired color.
Example: Using AccentColor in SwiftUI
import SwiftUI
@main
struct SemanticColorsApp: App {
var body: some Scene {
WindowGroup {
ContentView()
.accentColor(.purple) // Set accent color for the entire app
}
}
}
struct ContentView: View {
var body: some View {
VStack {
Text("Accent Color Example")
.foregroundStyle(Color.accentColor)
.padding()
.background(Color.accentColor.opacity(0.2))
.cornerRadius(10)
Button(action: {
print("Button tapped")
}) {
Text("Tap Me")
.padding()
.background(Color.accentColor)
.foregroundColor(.white)
.cornerRadius(10)
}
}
.padding()
}
}
In this example, we set the accent color of the entire app to purple using the accentColor
modifier. The Text
and Button
views use the accent color to create a consistent look.
Example: Using Primary and Secondary Colors
The primary
and secondary
colors are used for primary and secondary content, respectively. These colors adapt to the system’s color scheme and user settings.
Example: Using Primary and Secondary Colors in SwiftUI
import SwiftUI
struct PrimarySecondaryColorsView: View {
var body: some View {
VStack(spacing: 20) {
Text("Primary Color Example")
.foregroundStyle(Color.primary)
.padding()
.background(Color.primary.opacity(0.2))
.cornerRadius(10)
Text("Secondary Color Example")
.foregroundStyle(Color.secondary)
.padding()
.background(Color.secondary.opacity(0.2))
.cornerRadius(10)
Button(action: {
print("Primary Button tapped")
}) {
Text("Primary Button")
.padding()
.background(Color.primary)
.foregroundColor(.white)
.cornerRadius(10)
}
Button(action: {
print("Secondary Button tapped")
}) {
Text("Secondary Button")
.padding()
.background(Color.secondary)
.foregroundColor(.white)
.cornerRadius(10)
}
}
.padding()
}
}
In this example, we use Color.primary
and Color.secondary
to style text and buttons. These colors adapt to the system’s light and dark modes, providing a consistent and accessible user experience.
Example: Customizing System Colors
In addition to predefined semantic colors, you can customize colors in SwiftUI to create a unique look for your app. Custom colors can be defined in the asset catalog or directly in code.
Example: Using Custom Colors
import SwiftUI
struct CustomColorsView: View {
var body: some View {
VStack(spacing: 20) {
Text("Custom Color Example")
.foregroundStyle(Color("CustomColor"))
.padding()
.background(Color("CustomColor").opacity(0.2))
.cornerRadius(10)
Button(action: {
print("Custom Color Button tapped")
}) {
Text("Custom Color Button")
.padding()
.background(Color("CustomColor"))
.foregroundColor(.white)
.cornerRadius(10)
}
}
.padding()
}
}
To use custom colors:
- Open your asset catalog (Assets.xcassets).
- Create a new color set and name it
CustomColor
. - Choose your desired color.
In this example, we define a custom color in the asset catalog and use it in the Text
and Button
views.
Conclusion
Using semantic colors in SwiftUI allows you to create visually consistent and accessible user interfaces. By leveraging accentColor
, primary
, secondary
, and custom colors, you can ensure your app looks great across different themes and user settings.