SwiftUI offers a variety of semantic colors that adapt to different contexts and system settings,…
SecureField in SwiftUI
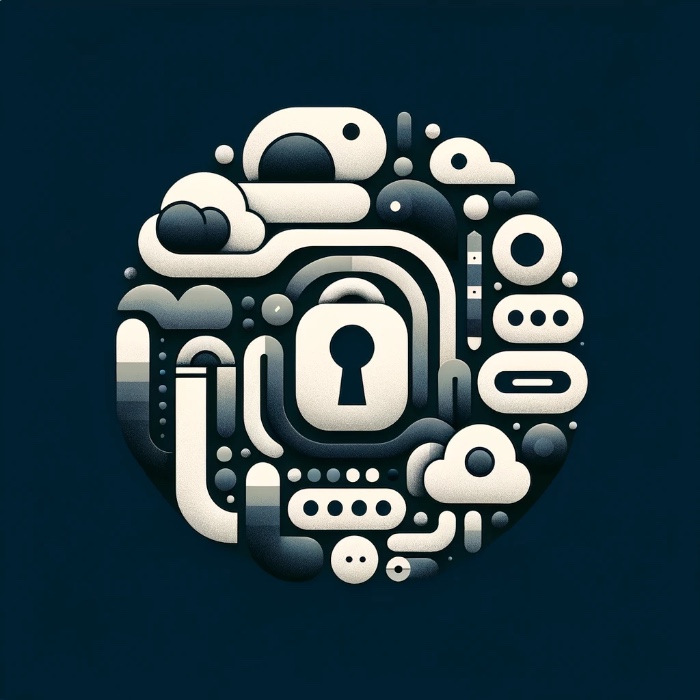
In this tutorial, we will explore how to use SecureField
in SwiftUI, which is designed for entering passwords or other sensitive information securely. SecureField
behaves similarly to a TextField
but masks the input to protect privacy. We’ll create a simple login form that uses both TextField
for the username and SecureField
for the password.
Step 1: Setting Up the Environment
First, let’s set up our SwiftUI view with state variables to hold the username and password:
import SwiftUI
struct LoginFormView: View {
@State private var username: String = ""
@State private var password: String = ""
}
Step 2: Creating the User Interface
Next, we will add a TextField
for the username input and a SecureField
for the password input within a form. We’ll ensure that the text input does not auto-capitalize or auto-correct, which is common for login forms.
var body: some View {
VStack {
Text("Current username: \(username)")
Text("Current password: \(password)")
TextField("Username", text: $username)
.autocorrectionDisabled(true)
.textInputAutocapitalization(.never)
.textFieldStyle(RoundedBorderTextFieldStyle())
.padding()
SecureField("Password", text: $password)
.textFieldStyle(RoundedBorderTextFieldStyle())
.padding()
}
.padding()
}
In this snippet, TextField
and SecureField
are embedded in a VStack
. The SecureField
masks the input, showing dots instead of the actual characters typed by the user.
Step 3: Handling Submissions
To handle form submissions, let’s add an onSubmit
modifier to SecureField
. This will trigger when the user presses the Return key after entering their password. We’ll define a handleLogin
method to process the login credentials.
SecureField("Password", text: $password)
.textFieldStyle(RoundedBorderTextFieldStyle())
.padding()
.onSubmit {
handleLogin()
}
func handleLogin() {
// Placeholder for login logic
print("Login attempted with username: \(username) and password: \(password)")
}
This setup calls handleLogin
when the form is submitted, allowing you to add whatever login logic your app requires.
Step 4: Enhancing User Guidance
Lastly, it’s often helpful to guide users with placeholder text. Here, we use the prompt as a placeholder to instruct users what to enter.
TextField("Username", text: $username, prompt: Text("Enter your username"))
SecureField("Password", text: $password, prompt: Text("Enter your password"))
Conclusion
SecureField
is a critical component in SwiftUI for handling sensitive information securely. By pairing it with TextField
for non-sensitive data, you can create forms that are both user-friendly and secure. This example demonstrates how to set up a basic login form, but you can expand upon this with additional security measures and UI enhancements as needed.