SwiftUI offers a variety of semantic colors that adapt to different contexts and system settings,…
Search in Your SwiftUI App
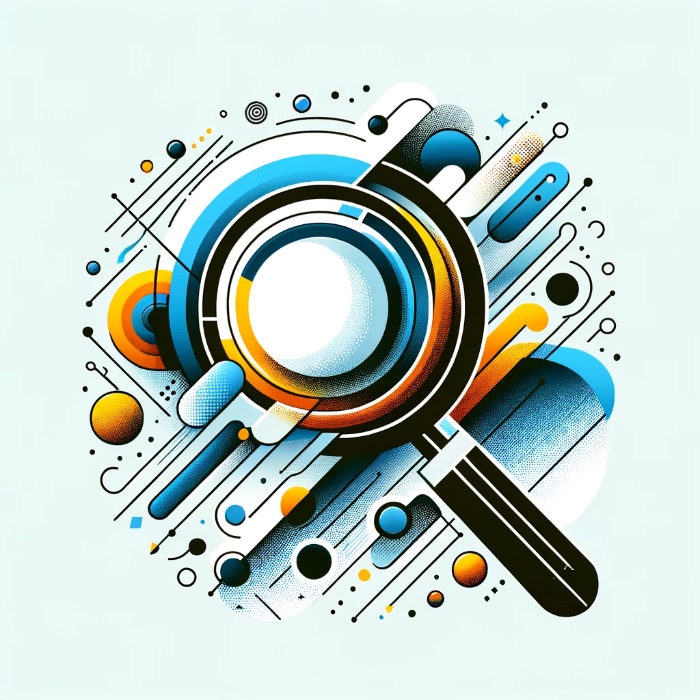
Creating a search feature in your SwiftUI app enhances user experience by making content easily discoverable. SwiftUI’s searchable
modifier simplifies integrating search functionality into your apps. Let’s explore a unique tutorial that illustrates adding a search interface with examples for various use cases.
Basic Search Interface
Let’s start with the most straightforward application of the searchable
modifier. We’ll add a search bar to a list view, allowing users to filter items based on their search queries.
Example: Simple Search in a List
import SwiftUI
struct SimpleSearchView: View {
let items = ["Apple", "Banana", "Orange", "Pear"]
@State private var searchText = ""
var filteredItems: [String] {
if searchText.isEmpty {
return items
} else {
return items.filter { $0.contains(searchText) }
}
}
var body: some View {
NavigationStack {
List(filteredItems, id: \.self) { item in
Text(item)
}
.searchable(text: $searchText)
.navigationTitle("Fruits")
}
}
}
This example demonstrates adding a search bar to a list of fruits. The list updates based on the user’s search input, showing filtered results.
Advanced Search with Custom Placement
SwiftUI allows for more control over the search interface’s placement, making it possible to tailor the search experience further.
Example: Search with Custom Placement
struct CustomPlacementSearchView: View {
let allItems = ["Apple", "Banana", "Orange", "Pear", "Mango", "Grapes"]
@State private var searchText = ""
var filteredItems: [String] {
if searchText.isEmpty {
return allItems
} else {
return allItems.filter { $0.localizedCaseInsensitiveContains(searchText) }
}
}
var body: some View {
NavigationStack {
List {
ForEach(filteredItems, id: \.self) { item in
Text(item)
}
}
.searchable(text: $searchText, placement:
.navigationBarDrawer(displayMode: .always))
.navigationTitle("Fruits")
}
}
}
Here, the search bar uses a custom placement in the navigation bar drawer, making it always visible and accessible.
Integrating Search in Complex Interfaces
In more complex interfaces, such as those with multiple columns or custom navigation structures, adding search functionality can enhance navigation and accessibility.
Example: Multi-Column Search Interface
struct MultiColumnSearchView: View {
let departments = ["Marketing", "Sales", "Engineering", "Design", "Customer Support"]
@State private var searchText = ""
var filteredDepartments: [String] {
if searchText.isEmpty {
return departments
} else {
return departments.filter { $0.localizedCaseInsensitiveContains(searchText) }
}
}
var body: some View {
NavigationSplitView {
List(filteredDepartments, id: \.self) { department in
NavigationLink(department, destination: MultiColumnSearchViewDetailView(department: department))
}
.searchable(text: $searchText)
.navigationTitle("Departments")
} detail: {
Text("Select a department")
}
}
}
struct MultiColumnSearchViewDetailView: View {
var department: String
var body: some View {
Text("\(department) details go here.")
.navigationTitle(department)
}
}
This setup illustrates adding a search bar within a NavigationSplitView, allowing users to search through the navigation links in a multi-column layout.
Conclusion
SwiftUI’s searchable modifier provides a flexible and powerful way to add search interfaces to your apps. By understanding and applying different configurations and placements, you can create intuitive and accessible search experiences for your users. Experiment with the examples provided and explore the possibilities to enhance your SwiftUI apps with effective search functionalities.