SwiftUI offers a variety of semantic colors that adapt to different contexts and system settings,…
Safe Areas in SwiftUI
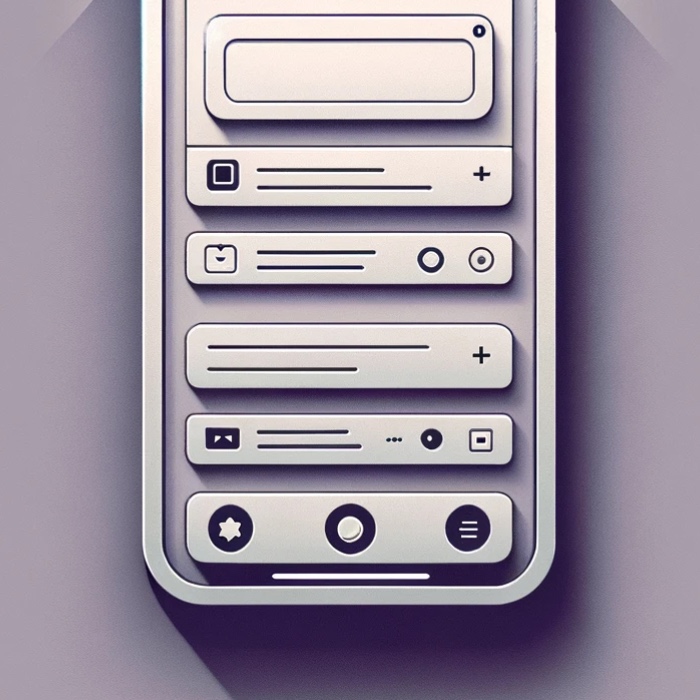
Safe areas are areas of a screen not covered by the status bar, notch, home indicator, or other overlays. In SwiftUI, respecting these areas ensures your content is visible and accessible, providing a seamless user experience. This post will comprehensively explore edgesIgnoringSafeArea()
, ignoresSafeArea()
, and safeAreaInset
, providing best practices and practical examples for each.
Safe Area Basics
When you create a view in SwiftUI, it automatically respects the safe area. However, you might sometimes want to extend your view behind these areas for visual effects, like background colors or images.
The edgesIgnoringSafeArea()
Modifier
edgesIgnoringSafeArea()
is used to extend the view under safe areas.
Example:
ZStack {
Color.green.edgesIgnoringSafeArea(.all)
Text("Hello, SwiftUI!")
}
In this example, the green background extends under all safe areas, but the text remains within the safe area.
The ignoresSafeArea()
Modifier
ignoresSafeArea()
is the newer, more flexible way to achieve
the same effect as edgesIgnoringSafeArea()
, but with added capabilities.
ZStack {
Color.blue
.ignoresSafeArea(edges: .top)
Text("Welcome to SwiftUI")
}
Here, the blue background ignores the safe area only at the top of the screen, allowing the view to extend under the status bar.
The safeAreaInset(edge:content:)
Modifier
safeAreaInset
allows you to add additional space in specific safe areas, which is useful for customizing layout behavior in response to safe area insets.
ScrollView {
VStack {
ForEach(1..<50) { index in
Text("Item \(index)")
}
}
}.safeAreaInset(edge: .bottom) {
Text("Bottom Text")
.frame(maxWidth: .infinity)
.padding(.top)
.background(.red)
}
In this example, a red bar is added at the bottom.
Best Practices for Using Safe Areas
- Understand the Context: Use safe area modifiers appropriately depending on the design requirements. Overriding safe areas can lead to content being hidden under system UI elements.
- Device Compatibility: Test your layout on different devices, including those with notches and home indicators, to ensure a consistent user experience.
- Accessibility Considerations: Always keep accessibility in mind. Overlapping content with system UI might hinder accessibility features.
- Use
ignoresSafeArea()
for Modern Code: Prefer usingignoresSafeArea()
as it is more versatile and future-proof compared toedgesIgnoringSafeArea()
. - Experiment with Previews: Utilize SwiftUI’s preview tool to test how your views behave with different safe area configurations.
Conclusion
Understanding and effectively using safe areas in SwiftUI is crucial for creating interfaces that look great and function well across all Apple devices. By following the guidelines and examples in this guide, developers can ensure their apps respect system UI elements while providing an immersive user experience.