SwiftUI offers a variety of semantic colors that adapt to different contexts and system settings,…
Observer Pattern SwiftUI
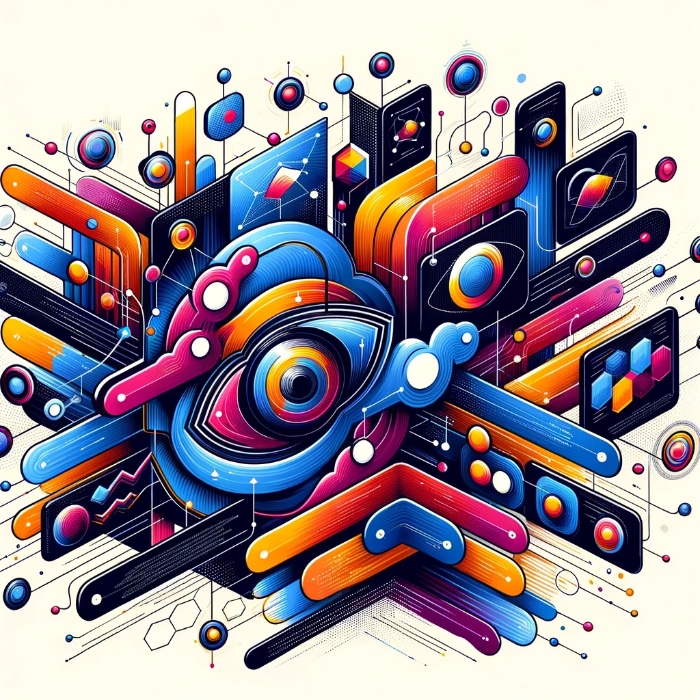
In the realm of software development, particularly within the SwiftUI framework, understanding and implementing design patterns can significantly elevate the structure and functionality of your applications. Among these, the Observer pattern stands out for its ability to streamline data flow and synchronization across different components of an app. Let’s delve into how this pattern can be adeptly applied in SwiftUI, transforming the way app states and data changes are managed.
The Observer Pattern: A Digital Classroom
Visualize a digital classroom where notifications and updates are constantly shared. Just as students stay alert to the teacher’s announcements, in app development, certain components keep an eye on specific data or state changes to react accordingly. This scenario mirrors the essence of the Observer pattern – establishing a watchful relationship between objects, where one (the subject) notifies others (the observers) about changes in its state.
Practical Application in SwiftUI: BooksCounterViewModel
Consider a SwiftUI application designed to track the number of books in a library. Employing the Observer pattern allows the app to update its UI in real-time as new books are added to the collection.
Implementation Example:
@Observable class BooksCounterViewModel {
var booksCount: Int = 0
func addBook() {
booksCount += 1
}
}
struct ContentView: View {
let viewModel = BooksCounterViewModel()
var body: some View {
VStack {
Button("Add One More Book", action: {
viewModel.addBook()
}).padding(.bottom)
Text("\(viewModel.booksCount) books in the Library")
}
.padding()
}
}
In this example, BooksCounterViewModel acts as the subject, keeping track of the book count. The ContentView, serving as the observer, reflects changes to booksCount instantly, ensuring the UI remains accurate and up-to-date without manual intervention.
Learn more: App Design Patterns in SwiftUI