SwiftUI offers a variety of semantic colors that adapt to different contexts and system settings,…
Observable protocol in SwiftUI
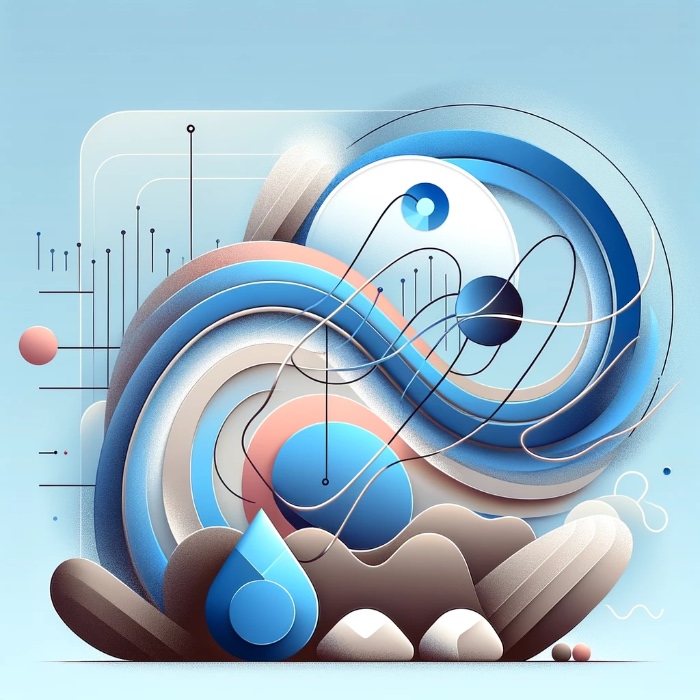
For this tutorial, we’ll explore the use of the @Observable
protocol in SwiftUI, focusing on an example that clearly demonstrates how you can utilize this powerful feature to create responsive applications. We’ll create a weather app scenario where various components of the weather data need to be observed for changes, influencing the app’s user interface dynamically.
Overview of @Observable in SwiftUI
@Observable
enables SwiftUI views to react to changes in data models by updating the UI automatically. This implementation follows the observer design pattern, which allows objects to be notified of changes in another object without tight coupling between them.
Step-by-Step Tutorial: Creating a Dynamic Weather Dashboard
Let’s build a simple weather app that updates its display based on changes to weather data.
Step 1: Define the Weather Data Model
First, we’ll define a WeatherData
class that will hold temperature, humidity, and weather condition information. We’ll make this class observable using @Observable
.
import SwiftUI
@Observable
class WeatherData {
var temperature: Double = 75.0
var humidity: Double = 50.0
var condition: String = "Sunny"
init(temperature: Double, humidity: Double, condition: String) {
self.temperature = temperature
self.humidity = humidity
self.condition = condition
}
}
Step 2: Create the Weather View
Now, we’ll create a WeatherView
that displays the temperature, humidity, and current weather condition. It will update these values whenever they change in the WeatherData
object.
struct WeatherView: View {
@Bindable var weatherData: WeatherData
var body: some View {
VStack {
Text("Current Temperature: \(weatherData.temperature)° F")
Text("Humidity: \(weatherData.humidity)%")
Text("Condition: \(weatherData.condition)")
Button("Refresh Weather") {
// Simulate receiving new weather data
weatherData.temperature = Double.random(in: 70...80)
weatherData.humidity = Double.random(in: 40...60)
weatherData.condition = ["Rainy", "Sunny", "Cloudy"].randomElement()!
}
}
.padding()
.background(Color.blue)
.foregroundColor(.white)
.clipShape(RoundedRectangle(cornerRadius: 10))
}
}
Step 3: Integrate Weather Data in App View
Finally, integrate the WeatherData
and WeatherView
into the main content view of the app.
struct ContentView: View {
var weatherData = WeatherData(temperature: 75, humidity: 50, condition: "Sunny")
var body: some View {
WeatherView(weatherData: weatherData)
}
}
Conclusion
This example shows how to use @Observable
in SwiftUI to build an app that responds dynamically to changes in its data model. The WeatherView
updates automatically as the WeatherData
changes, demonstrating a practical application of the observer design pattern without tight coupling between UI components and the data model. By using @Observable
, developers can ensure their apps remain responsive and the UI consistently reflects the current state of the application data.