SwiftUI offers a variety of semantic colors that adapt to different contexts and system settings,…
NavigationSplitView in SwiftUI
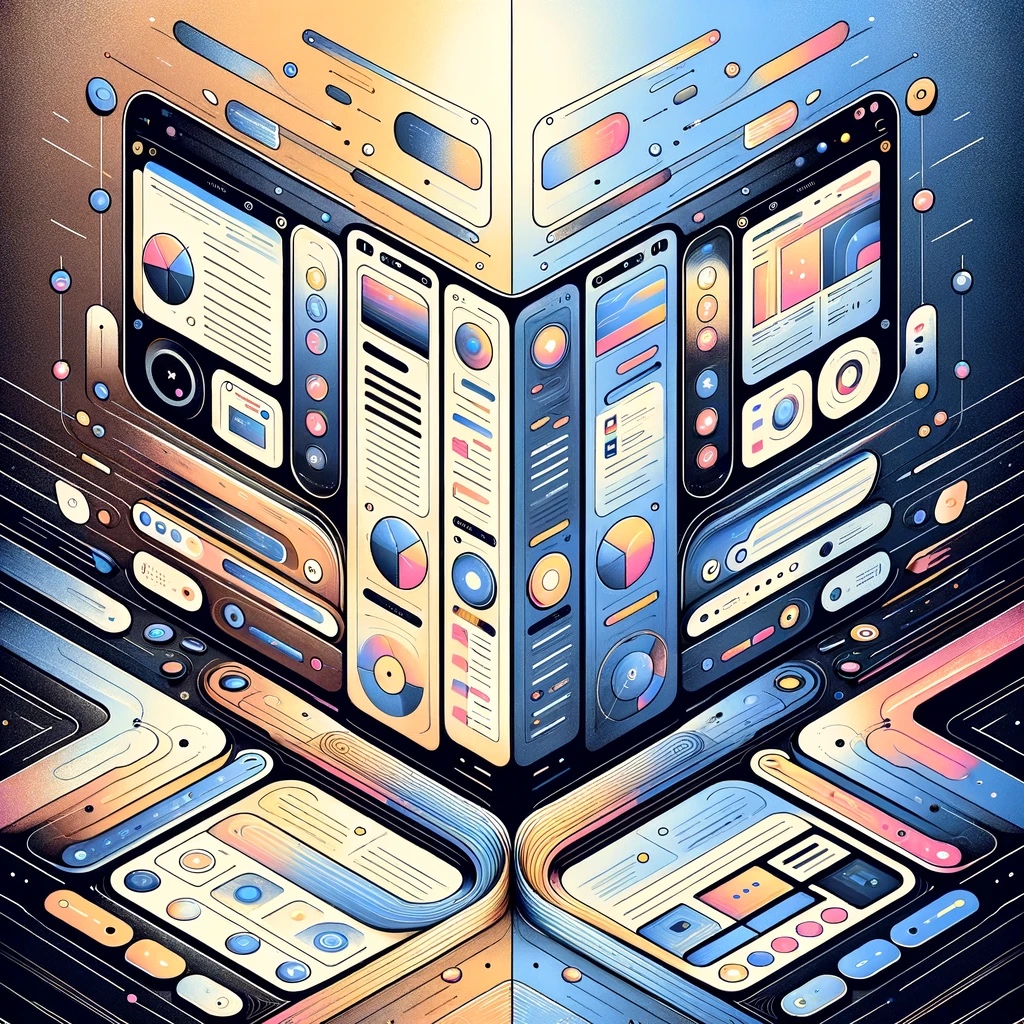
NavigationSplitView in SwiftUI is a versatile component introduced to create multi-column navigation interfaces that adapt across iOS, iPadOS, macOS, and other Apple platforms. Ideal for crafting master-detail layouts, NavigationSplitView
enables developers to construct applications that efficiently utilize available screen space, presenting information in a more organized and accessible manner. In this tutorial, we’ll walk through implementing a simple two-column navigation example that lists subjects and displays their details upon selection.
What is NavigationSplitView?
NavigationSplitView
offers a straightforward way to build adaptive, multi-column UIs in SwiftUI applications. It can dynamically adjust its layout based on the device’s screen size and orientation, presenting information in two or three columns. On larger screens, it can display side-by-side columns, while on compact screens, it collapses into a single column, enhancing the app’s usability across different devices.
Tutorial: Implementing a Simple Two-Column Layout using NavigationSplitView
Step 1: Define Your Data Model
First, define a simple data model to represent the subjects. Each subject has a unique identifier (id
) and a name.
struct Subject: Identifiable, Hashable {
var id = UUID()
var name: String
}
Step 2: Prepare Sample Data
Create an array of subjects to be displayed in the list. This array serves as the data source for our two-column navigation interface.
let subjects = [Subject(name: "Subject 1"), Subject(name: "Subject 2")]
Step 3: Create the List View
Construct a view that lists the subjects. Each subject in the list can be tapped, updating the current selection.
struct SubjectListView: View {
let subjects: [Subject]
@Binding var selectedSubject: Subject?
var body: some View {
List(subjects) { subject in
Button(subject.name) {
self.selectedSubject = subject
}
}
}
}
Step 4: Design the Detail View
The detail view displays information about the selected subject. If no subject is selected, it prompts the user to make a selection.
struct SubjectDetailView: View {
var subject: Subject?
var body: some View {
Text(subject?.name ?? "Select a subject")
}
}
Step 5: Combine Views with NavigationSplitView
Now, integrate the list and detail views using NavigationSplitView
. This setup automatically adapts the UI layout to the device’s screen size and orientation.
struct ContentView: View {
@State private var selectedSubject: Subject?
@State private var columnVisibility: NavigationSplitViewVisibility = .all
var body: some View {
NavigationSplitView(columnVisibility: $columnVisibility) {
List(subjects, id: \.id, selection: $selectedSubject) { subject in
Text(subject.name)
.font(.title)
.onTapGesture {
self.selectedSubject = subject
}
}
} detail: {
if let selectedSubject = selectedSubject {
SubjectDetailView(subject: selectedSubject)
} else {
Text("Select a subject")
}
}
.navigationSplitViewStyle(.balanced)
}
}
Conclusion
By following these steps, you’ve created a responsive, two-column navigation interface using SwiftUI’s NavigationSplitView
. This layout adapts seamlessly across different devices, providing an intuitive user experience whether on an iPhone, iPad, or Mac. Experiment with customizing the views, adapting the data model, and exploring additional NavigationSplitView
features to further enhance your SwiftUI applications.