SwiftUI offers a variety of semantic colors that adapt to different contexts and system settings,…
NavigationBarItem in SwiftUI
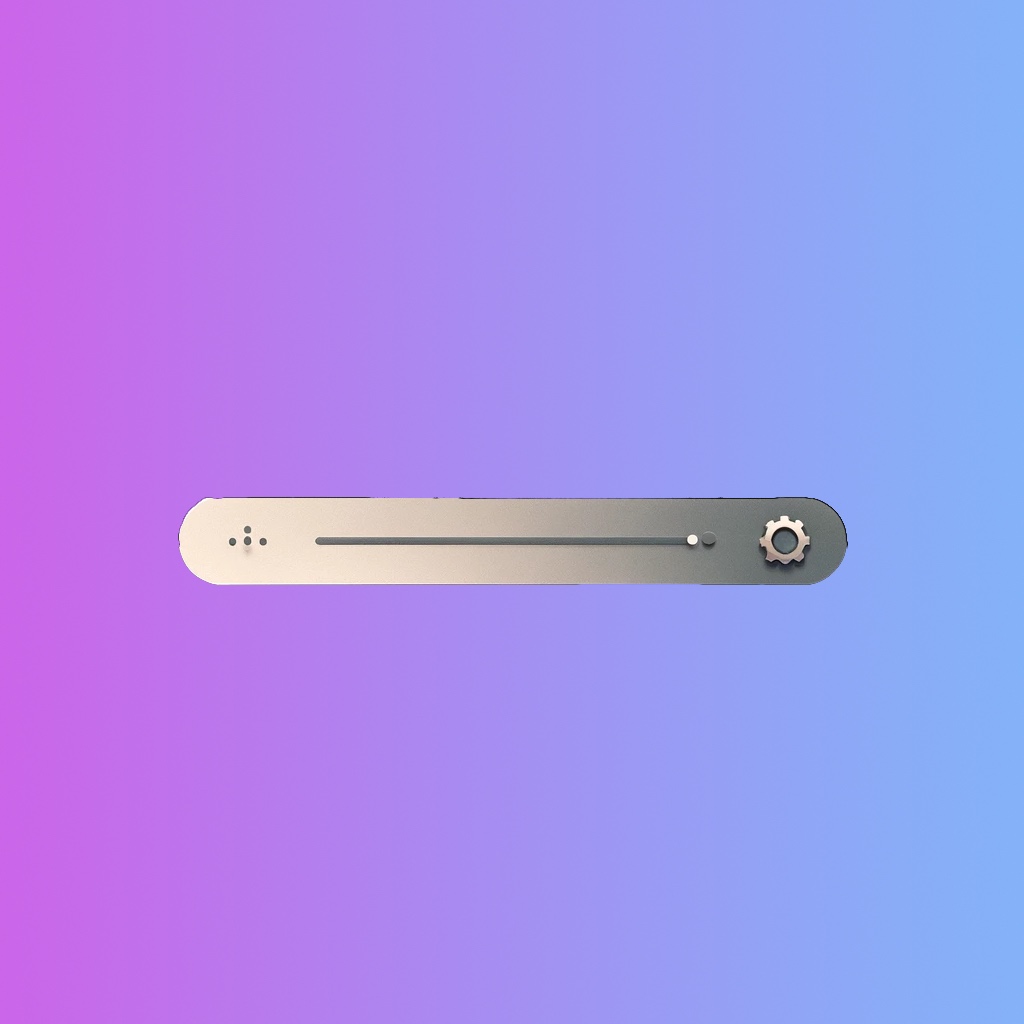
In SwiftUI, a NavigationBarItem is used within a navigation bar to provide interactive elements—such as buttons or custom views—that facilitate navigation or perform actions. Proper use of NavigationBarItem enhances user experience by making app navigation seamless and intuitive.
Step 1: Create a Navigation Stack first
import SwiftUI
struct NavigationBarItemSwiftUI: View {
var body: some View {
NavigationStack {
Text("Welcome to SwiftUI Navigation")
.navigationTitle("Home")
}
}
}
#Preview {
ContentView()
}
Step 2: Add Navigation Bar Items
Now, let’s add a NavigationBarItem
to our navigation bar. SwiftUI allows us to add items to the leading or trailing edge of the navigation bar.
Adding a Leading Navigation Item
.navigationBarItems(leading:
Button(action: {
print("Leading item tapped")
}) {
Image(systemName: "bell")
}
)
Adding a Trailing Navigation Item
.navigationBarItems(trailing:
Button(action: {
print("Trailing item tapped")
}) {
Image(systemName: "gear")
}
)
Step 3: Customizing Navigation Bar Items
You can also customize elements inside your navigation bar
.navigationBarItems(trailing:
Button(action: {
// Action
print("Edit Button Tapped")
}) {
Text("Edit")
.foregroundColor(.blue)
.font(.headline)
}
)
Best Practices
When using NavigationBarItem
in your apps, consider the following best practices to enhance usability:
- Keep navigation bar items minimal to avoid clutter.
- Use universally recognized icons for common actions.
- Ensure that tap targets are appropriately sized for touch interactions.
Conclusion
NavigationBarItem
in SwiftUI is a powerful tool for adding navigation and action items to your app’s navigation bar. By following this guide, you’ll be well on your way to creating more navigable and user-friendly SwiftUI apps.