SwiftUI offers a variety of semantic colors that adapt to different contexts and system settings,…
MultiDatePicker in SwiftUI
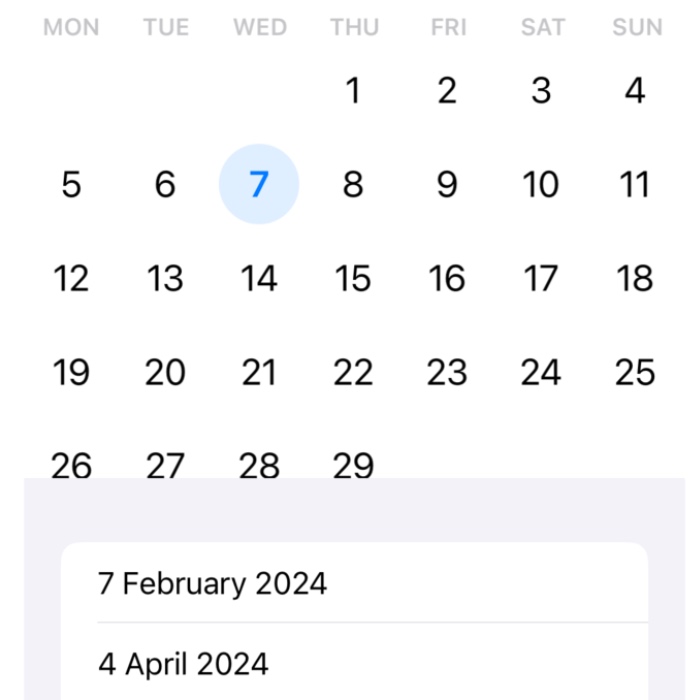
A MultiDatePicker
in SwiftUI allows users to select multiple dates, which can be extremely useful for applications such as event planners, task schedulers, or any scenario where date range selection is necessary. In this tutorial, we’ll walk through creating a unique example of a MultiDatePicker
that helps users select dates for a vacation planning app.
Using MultiDatePicker – Simple Vacation Tracker example
In this tutorial, we will explore how to leverage MultiDatePicker
to build a user-friendly vacation tracker app. This app will not only help users plan their holidays but also visualize them in a compelling way.
Begin by setting up your SwiftUI view’s structure:
import SwiftUI
struct VacationTrackerView: View {
@State private var selectedDates: Set<DateComponents> = []
var body: some View {
NavigationView {
VStack {
multiDatePicker
selectedDatesList
}
.navigationTitle("Vacation Tracker")
.padding()
}
}
private var multiDatePicker: some View {
MultiDatePicker(
"Plan Your Vacations",
selection: $selectedDates,
displayedComponents: [.date] // Show only the date component
)
.frame(height: 300)
}
private var selectedDatesList: some View {
List(selectedDates.sorted(by: { $0.date! < $1.date! }), id: \.self) { date in
Text("\(date.date!, formatter: itemFormatter)")
}
}
}
// Helper to format the date
private let itemFormatter: DateFormatter = {
let formatter = DateFormatter()
formatter.dateStyle = .long
return formatter
}()
This SwiftUI tutorial introduces you to the MultiDatePicker
component, a valuable addition for any developer looking to streamline date selection in their apps. By building a vacation tracker, you learn to integrate multiple date selections with other UI elements, enhancing both functionality and aesthetics.