SwiftUI offers a variety of semantic colors that adapt to different contexts and system settings,…
Mastering Canvas, GraphicsContext, and Styles in SwiftUI
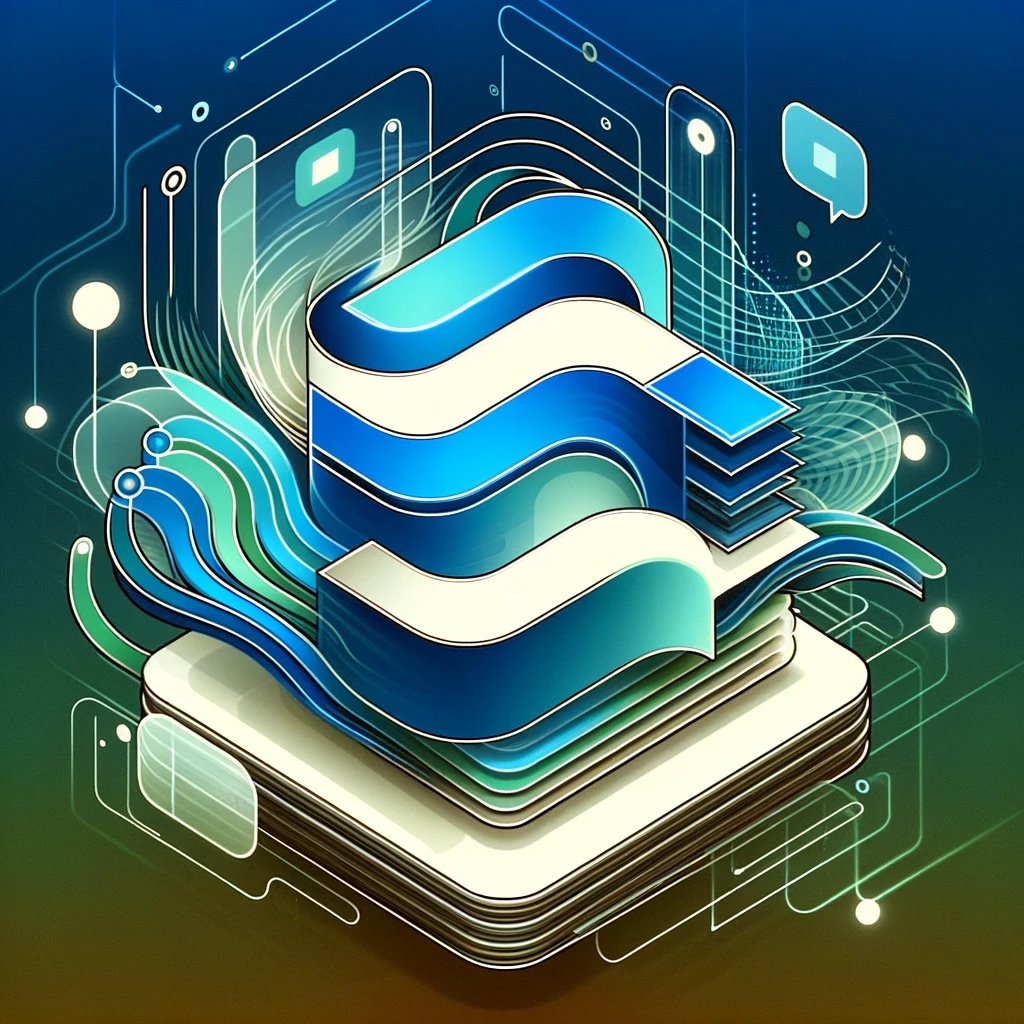
SwiftUI offers a rich set of tools for creating and customizing user interfaces. Among these are Canvas
, GraphicsContext
, Border
, ForegroundStyle
, and BackgroundStyle
. This tutorial will guide you through the basics of these powerful features with unique and easy-to-follow examples.
Introduction to Canvas
Canvas
is a view in SwiftUI that provides a drawing area for creating custom graphics. It uses GraphicsContext
to draw shapes, images, and text.
Example: Drawing with Canvas and GraphicsContext
import SwiftUI
struct CanvasDrawingView: View {
var body: some View {
Canvas { context, size in
context.draw(Text("Hello, SwiftUI!"), at: CGPoint(x: size.width / 2, y: size.height / 2))
let circle = Path(ellipseIn: CGRect(x: size.width / 4, y: size.height / 4, width: size.width / 2, height: size.height / 2))
context.fill(circle, with: .color(.blue))
}
.frame(width: 300, height: 300)
.border(Color.black, width: 2)
}
}
In this example, we use Canvas
to draw text and a blue circle. The GraphicsContext
allows us to draw shapes and text at specific positions within the canvas.
Using Border
The border
modifier adds a border around a view. You can customize the border’s color, width, and style.
Example: Applying a Border to a View
struct BorderedView: View {
var body: some View {
Text("Bordered Text")
.padding()
.border(Color.red, width: 4)
}
}
In this example, we add a red border around a text view.
ForegroundStyle
ForegroundStyle
allows you to set the style for the foreground content of a view, such as text or shapes.
Example: Using ForegroundStyle with Text
struct ForegroundStyledView: View {
var body: some View {
Text("Stylized Text")
.font(.largeTitle)
.foregroundStyle(
LinearGradient(
gradient: Gradient(colors: [.red, .orange]),
startPoint: .leading,
endPoint: .trailing
)
)
.padding()
}
}
In this example, we use a linear gradient as the foreground style for the text, creating a smooth color transition.
BackgroundStyle
BackgroundStyle
allows you to set the background style of a view.
struct BackgroundStyledView: View {
var body: some View {
Text("Stylized Background")
.padding()
.background(
LinearGradient(
gradient: Gradient(colors: [.blue, .purple]),
startPoint: .top,
endPoint: .bottom
)
)
.cornerRadius(10)
.padding()
}
}
In this example:
- We apply a
LinearGradient
as the background to aText
view using the.background
modifier. - The gradient transitions from blue at the top to purple at the bottom.
- We add a corner radius to the text view to give it rounded corners.
Conclusion
SwiftUI’s Canvas
, GraphicsContext
, Border
, ForegroundStyle
, and BackgroundStyle
provide powerful tools for creating custom graphics and styling views. By understanding and using these features, you can enhance the visual appeal and functionality of your SwiftUI applications.