SwiftUI offers a variety of semantic colors that adapt to different contexts and system settings,…
Localizing Text in SwiftUI with LocalizedStringKey
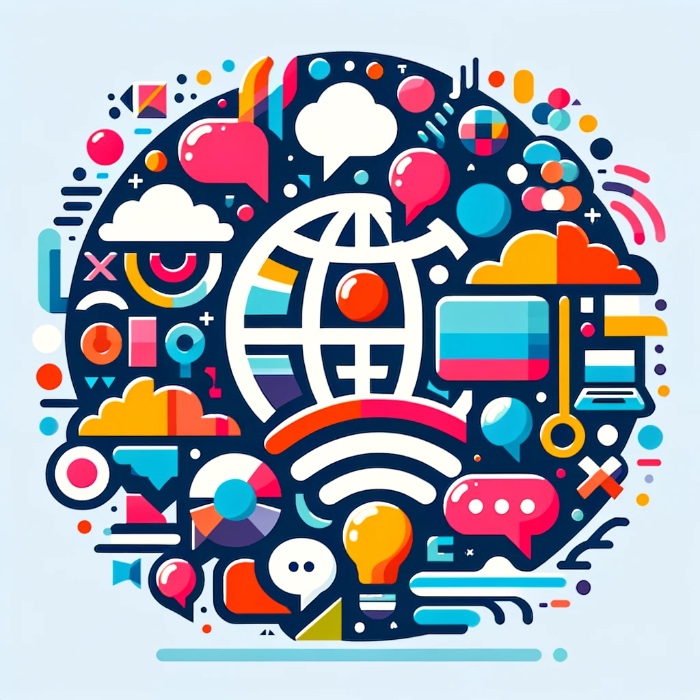
In SwiftUI, localization plays a crucial role in making your app accessible and user-friendly across various languages and regions. The LocalizedStringKey
struct is a powerful tool for achieving seamless localization by allowing developers to manage localized strings effectively.
What is LocalizedStringKey?
LocalizedStringKey
is a type used to retrieve localized strings from .strings files within your app’s bundle. When you use string literals in SwiftUI elements like Text
, Toggle
, or Picker
, SwiftUI automatically uses LocalizedStringKey
to fetch the appropriate localized version of the string. This automatic mechanism is possible because LocalizedStringKey
conforms to the ExpressibleByStringLiteral
protocol.
Localized and Non-Localized Text
The beauty of LocalizedStringKey
lies in its flexibility. For instance, when you initialize a Text
view with a string literal, SwiftUI implicitly converts this string into a LocalizedStringKey
and looks up the localized string:
Text("Welcome")
In contrast, if you pass a String
variable to the initializer, SwiftUI treats this as a direct instruction to use the string as-is, without localization. This approach is particularly useful when displaying dynamic content, such as user-generated data, that doesn’t require localization:
let dynamicText = "User's input text"
Text(dynamicText)
However, there are scenarios where you might need to localize a string stored in a variable. In such cases, you can explicitly convert your string into a LocalizedStringKey
to ensure it is localized:
let greeting = "Hello"
Text(LocalizedStringKey(greeting))
Practical Example
Imagine a simple task list app that supports multiple languages. You want the static text to be localized, but the task descriptions, which are user input, should not be:
struct TaskListView: View {
let tasks = ["Buy milk", "Schedule meeting", "Call mom"]
var body: some View {
List {
Text("Tasks for today").fontWeight(.bold) // This will be localized
ForEach(tasks, id: \.self) { task in
Text(task) // Displays user input directly
}
}
}
}
If your app supports German, for example, and your Localizable.strings
file contains:
“Tasks for today” = “Aufgaben für heute”;
The header “Tasks for today” will appear as “Aufgaben für heute” to German users, enhancing the localized experience while keeping the task descriptions in the original language provided by the user.
Conclusion
LocalizedStringKey
enhances SwiftUI’s text handling capabilities by providing a simple yet effective way to support multiple languages in your app. By distinguishing between when to use string literals and when to use string variables, you can control the localization behavior of your app, making it intuitive and accessible to a global audience. Embrace LocalizedStringKey
in your SwiftUI projects to seamlessly integrate localization and improve user engagement worldwide.