SwiftUI offers a variety of semantic colors that adapt to different contexts and system settings,…
List in SwiftUI
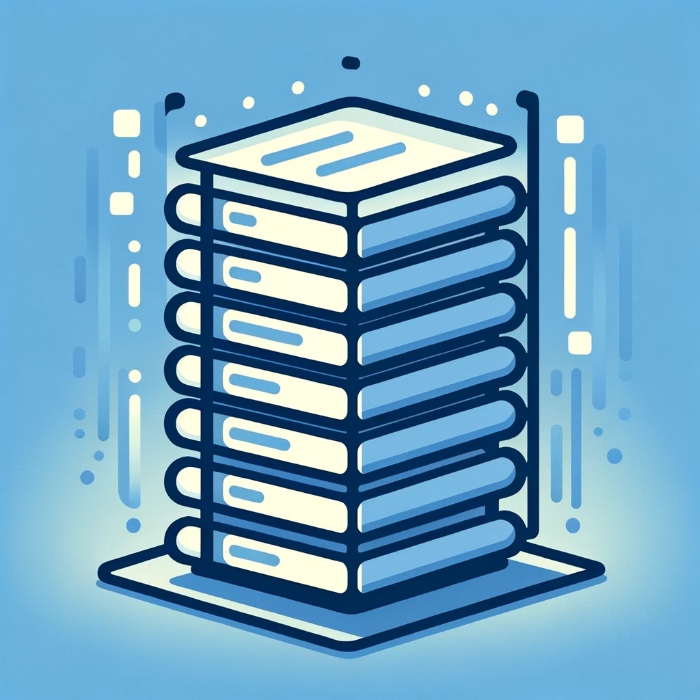
In SwiftUI, a List is a container that displays rows of data arranged in a single column. It’s similar to UITableView in UIKit but with a more straightforward, declarative syntax. Having a collection of items in a List is common in various apps.
Static List – Creating a basic, static list.
import SwiftUI
struct ContentView: View {
var body: some View {
List {
Text("Item 1")
Text("Item 2")
Text("Item 3")
}
}
}
This code creates a list with three static text items.
Using ForEach with List
ForEach is used in SwiftUI to iterate over a collection and create views for each item. It’s commonly used within a List to display dynamic data. Suppose you have an array of strings. You can use ForEach to create a list item for each string.
struct ContentView: View {
let items = ["Apple", "Banana", "Cherry"]
var body: some View {
List {
ForEach(items, id: \.self) { item in
Text(item)
}
}
}
}
Each item in the items array is represented as a row in the list.
ForEach with List Benefits
- Flexibility: This method allows you to mix different types of items within the same list. You can include static views alongside your dynamic
ForEach
content. - More Control: It gives you more control over the behavior of individual list items. For instance, you can apply modifiers to specific elements or use different types of views within the same
List
. - Advanced Functionality: You can use
onDelete
andonMove
modifiers withForEach
for functionalities like deletion and reordering of list items.
ForEach with List Use Cases:
- When you need a list with a mix of static and dynamic content.
- When the list requires more complex interactions, like swipe to delete or drag to reorder.
- When you want to apply different modifiers or styles to different rows.
This is how the code will look like without using ForEach
struct ContentView: View {
let items = ["Apple", "Banana", "Cherry"]
var body: some View {
List(items, id: \.self) { item in
Text(item)
}
}
}
List without ForEach Benefits
- Simplicity and Conciseness: This is a more concise way to create a list. SwiftUI internally uses
ForEach
to iterate over the items, so it’s simpler and more straightforward. - Limited to Dynamic Content: This approach is limited to displaying dynamic content. You can’t mix in static views as easily as with the
ForEach
method. - Reduced Control: There’s less control over individual items compared to using
ForEach
explicitly.
List without ForEach Use Cases:
- Ideal for simple lists where each item is of the same type and requires similar treatment.
- Best suited for displaying a straightforward collection without the need for additional list functionalities like deletion or reordering.
- Useful when you prefer brevity and simplicity in your code for straightforward tasks.
The choice between these two approaches depends on the requirements of your specific use case. If you need a simple list without complex functionalities, go for the direct List(items, id: \.self)
approach. However, if you require more control, mixed content types, or additional functionalities like deletion and reordering, List { ForEach(...) }
is the way to go.
Properties of List
List in SwiftUI comes with various properties that allow you to customize its appearance and behavior.
Here are some commonly used properties:
listStyle
: Defines the style of the list, like grouped or plain.onDelete
: Adds the ability to delete rows.onMove
: Enables row reordering.
.listStyle – Customizing List
The listStyle
modifier allows you to change the appearance of your list. SwiftUI offers several styles, such as PlainListStyle
, GroupedListStyle
, and more.
struct ContentView: View {
var body: some View {
List {
Text("Item 1")
Text("Item 2")
Text("Item 3")
}
.listStyle(GroupedListStyle())
}
}
In this example, the GroupedListStyle
gives the list a distinct grouped appearance, which is commonly used in settings or contacts apps.
.onDelete – List Row Deletion
The onDelete
modifier allows users to delete rows from the list, typically by swiping left on a row.
struct ContentView: View {
@State private var items = ["Apple", "Banana", "Cherry"]
var body: some View {
List {
ForEach(items, id: \.self) { item in
Text(item)
}
.onDelete(perform: deleteItems)
}
}
func deleteItems(at offsets: IndexSet) {
items.remove(atOffsets: offsets)
}
}
In this code, onDelete
calls the deleteItems
function, which removes the item at the swiped index.
.onMove – Reordering List Rows
The onMove
modifier enables users to reorder rows in the list.
struct ContentView: View {
@State private var items = ["Apple", "Banana", "Cherry"]
var body: some View {
NavigationView {
List {
ForEach(items, id: \.self) { item in
Text(item)
}
.onMove(perform: moveItems)
}
.toolbar {
EditButton()
}
}
}
func moveItems(from source: IndexSet, to destination: Int) {
items.move(fromOffsets: source, toOffset: destination)
}
}
Here, onMove
is used with an EditButton
in a toolbar
. The moveItems
function rearranges the items when the user drags a row to a new position.
ScrollView vs. List
While ScrollView
and List
might seem similar, they serve different purposes. ScrollView
is a generic container that can hold any content and make it scrollable. List
, on the other hand, is specifically tailored for displaying rows of data, particularly when the data is dynamic or extensive.
Use ScrollView when you need more control over the layout or when you are not dealing with a standard list of items.
ScrollView {
VStack {
Text("Content 1")
Text("Content 2")
}
}
Use Cases of List in Your App
- Displaying a dynamic list of items, like a list of messages or contacts.
- Showing settings or options where each item navigates to a different view.
- Creating to-do lists or checklists
Conclusion
List
in SwiftUI is an incredibly powerful and versatile UI component, suitable for a wide range of applications. Its integration with ForEach
makes it easy to display dynamic data, while its various properties allow for significant customization. Understanding when to use List
versus ScrollView
is key in designing effective SwiftUI interfaces.