SwiftUI offers a variety of semantic colors that adapt to different contexts and system settings,…
Keyboard Types in SwiftUI
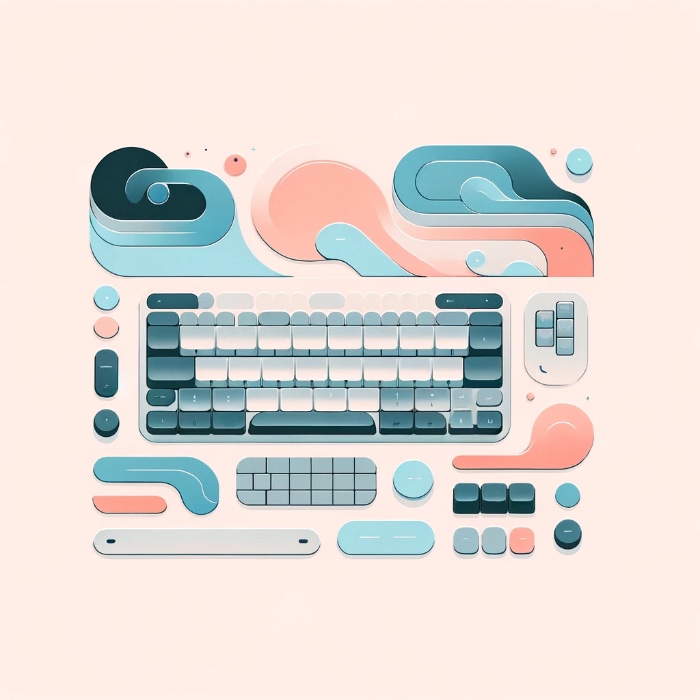
Creating user-friendly forms in SwiftUI apps often involves providing the appropriate keyboard for specific types of input. Understanding how to use various keyboard types can significantly enhance the user experience by making text entry easier and more intuitive. In this tutorial, we’ll explore how to set keyboard types for text fields in SwiftUI, ensuring that users encounter the right keyboard for the data they need to enter.
Understanding Keyboard Types in SwiftUI
SwiftUI allows developers to specify the keyboard type for text views, which tailors the keyboard layout to the expected content type, such as numbers, email addresses, or URLs. This is accomplished using the .keyboardType(_:)
modifier, which accepts a parameter from the UIKeyboardType
enumeration.
Example: Setting Up Various Text Fields with Specific Keyboard Types
Here’s a practical example demonstrating how to implement different keyboard types for a variety of text input needs in a SwiftUI view.
import SwiftUI
struct KeyboardTypeExampleView: View {
@State private var emailAddress: String = ""
@State private var phoneNumber: String = ""
@State private var url: String = ""
@State private var quantity: String = ""
var body: some View {
Form {
Section(header: Text("Contact Information")) {
TextField("Email Address", text: $emailAddress)
.keyboardType(.emailAddress)
.autocapitalization(.none)
TextField("Phone Number", text: $phoneNumber)
.keyboardType(.phonePad)
}
Section(header: Text("Website")) {
TextField("Enter URL", text: $url)
.keyboardType(.URL)
.autocapitalization(.none)
}
Section(header: Text("Order Quantity")) {
TextField("Quantity", text: $quantity)
.keyboardType(.numberPad)
}
}
}
}
Explanation of Keyboard Types Used
- Email Address: The
.emailAddress
keyboard is optimized for email entry, making it easier to access symbols commonly used in email addresses. - Phone Number: The
.phonePad
keyboard displays a numeric keypad, ideal for entering phone numbers. - URL: The
.URL
keyboard is tailored for URL entry, with convenience keys for symbols commonly used in web addresses. - Quantity: The
.numberPad
keyboard presents a simple numeric pad, perfect for entering numeric values such as quantities.
Best Practices for Keyboard Types
- Match the Keyboard to the Input Type: Always choose the keyboard layout that best matches the expected input to minimize user effort and potential input errors.
- Disable Auto-Capitalization Where Necessary: For fields like email addresses and URLs, disable auto-capitalization to prevent the automatic capitalization of the first letter.
- Use Appropriate Return Keys: You can also customize the return key on the keyboard to reflect the action the user will take, like “Search” or “Done”, which provides an additional clue about what happens next.
By carefully selecting the appropriate keyboard type for each text input field in your SwiftUI views, you can create a more streamlined, efficient, and user-friendly data entry experience.