SwiftUI offers a variety of semantic colors that adapt to different contexts and system settings,…
Grids in SwiftUI – LazyHGrid, LazyVGrid, GridItem, GridRow
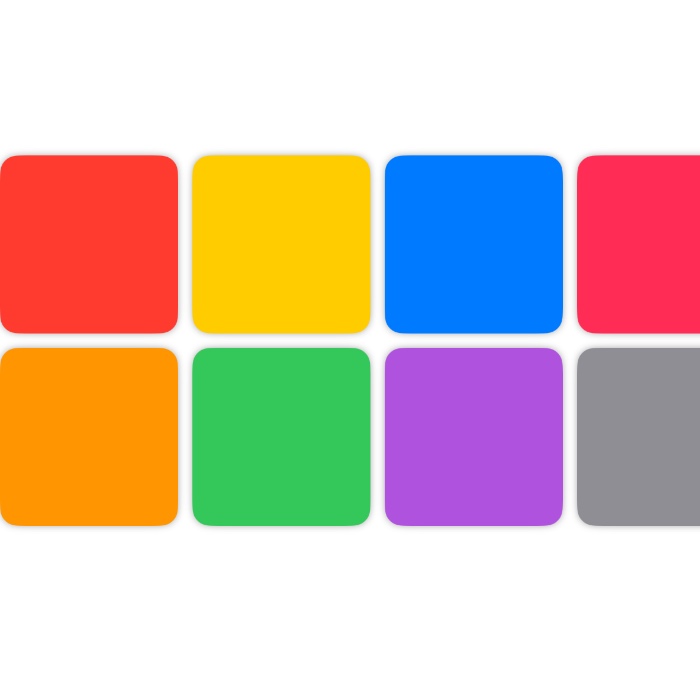
Creating grids in SwiftUI allows you to organize content in a structured, visually appealing layout, whether you’re building a gallery, a product list, or a custom user interface component. SwiftUI provides several grid views to accommodate different layout needs, including Grid
, LazyHGrid
, LazyVGrid
, and GridItem
. This tutorial will guide you through using these components to create responsive and dynamic grid layouts in your SwiftUI applications.
Overview of SwiftUI Grid Components
- LazyHGrid and LazyVGrid: These views are used for creating horizontal and vertical scrolling grids, respectively. They are “lazy” because they load the content on demand, which is efficient for displaying large collections of data.
- GridItem: Represents the configuration for items within a
LazyHGrid
orLazyVGrid
, allowing you to specify sizing behavior. - GridRow: This component is used within a
Grid
to define rows of items, managing layout in a more granular way.
Understanding Grids in SwiftUI: A Practical Example
Grids in SwiftUI offer a powerful solution for arranging content systematically. By using grids we can efficiently manage large collections of data with minimal performance overhead. Let’s dive into creating a simple app that utilizes grid components.
This SwiftUI example demonstrates how to create a simple, yet visually appealing two-column grid using the Grid
and GridRow
components. The grid dynamically displays a list of colors, organizing them into two columns for a cleaner and more structured layout.
struct SwiftUIGrids: View {
private let colors: [Color] = [.red, .orange, .yellow, .green, .blue, .purple, .pink, .gray, .black, .white]
var body: some View {
ScrollView {
// Header for columns
Grid {
GridRow {
Text("Column 1").bold().frame(maxWidth: .infinity)
Text("Column 2").bold().frame(maxWidth: .infinity)
}
// Rows containing actual colors, split into two columns
ForEach(0..<colors.count, id: \.self) { index in
if index % 2 == 0 { // Start new row for every two colors
GridRow {
if index < colors.count {
colorView(for: colors[index])
}
if index + 1 < colors.count {
colorView(for: colors[index + 1])
}
}
}
}
}
.padding(.horizontal)
}
}
/// View for a single color box
func colorView(for color: Color) -> some View {
color
.frame(width: 100, height: 100)
.cornerRadius(10)
.shadow(radius: 2)
.frame(maxWidth: .infinity)
}
}
LazyVGrid and LazyHGrid
In SwiftUI, LazyVGrid
and LazyHGrid
offer powerful and flexible ways to handle grid layouts that adapt efficiently to the content’s size and the device’s screen dimensions. They’re particularly useful when dealing with a large number of items that should not all be rendered immediately, which enhances performance by only loading items as needed.
LazyVGrid – Vertical Grid Layout
LazyVGrid
arranges its children in a vertical grid format. It is ideal for situations where you want to display multiple columns of content that can scroll vertically, managing a large dataset effectively. This grid type dynamically loads and unloads views as the user scrolls, which is optimal for memory management and smooth user experience.
Example with Color Palette: Using the previously discussed color palette grid, implementing it with LazyVGrid
would allow us to display a vertical grid of colors that loads dynamically. Users can scroll through the palette vertically, and the grid will only render visible items, improving performance for large color sets.
struct LazyVGridExample: View {
private let colors: [Color] = [.red, .orange, .yellow, .green, .blue, .purple, .pink, .gray, .black, .white]
private let columns = [
GridItem(.flexible()),
GridItem(.flexible())
]
var body: some View {
ScrollView {
LazyVGrid(columns: columns) {
ForEach(Array(colors.enumerated()), id: \.element) { index, color in
color
.frame(height: 100)
.cornerRadius(10)
.shadow(radius: 2)
}
}
.padding(.horizontal)
}
}
}
LazyHGrid – Horizontal Grid Layout
Conversely, LazyHGrid
arranges its children in a horizontal grid format. It is suited for displaying rows that can be scrolled horizontally. This is particularly useful in apps where horizontal space is limited, or where a horizontal scrolling mechanism enhances the user interface.
Example Usage: If we adapt our color palette to use LazyHGrid
, the palette will allow horizontal scrolling, accommodating scenarios where vertical space is constrained, such as in landscape mode on mobile devices.
struct LazyHGridExample: View {
private let colors: [Color] = [.red, .orange, .yellow, .green, .blue, .purple, .pink, .gray, .black, .white]
private let rows = [
GridItem(.fixed(100)),
GridItem(.fixed(100))
]
var body: some View {
ScrollView(.horizontal) {
LazyHGrid(rows: rows) {
ForEach(Array(colors.enumerated()), id: \.element) { index, color in
color
.frame(width: 100)
.cornerRadius(10)
.shadow(radius: 2)
}
}
.padding(.vertical)
}
}
}
Benefits of Lazy Grids
- Efficiency in Rendering: Lazy grids load content on demand, which makes them highly efficient, particularly for large collections of data.
- Flexibility: Both grid types offer the ability to adjust spacing, alignment, and the number of tracks via
GridItem
, providing significant flexibility in layout design. - Adaptive Layouts: With support for different screen sizes and orientations, these grids help create responsive and adaptive user interfaces.