SwiftUI offers a variety of semantic colors that adapt to different contexts and system settings,…
Gradients in SwiftUI – Linear, Radial, Angular
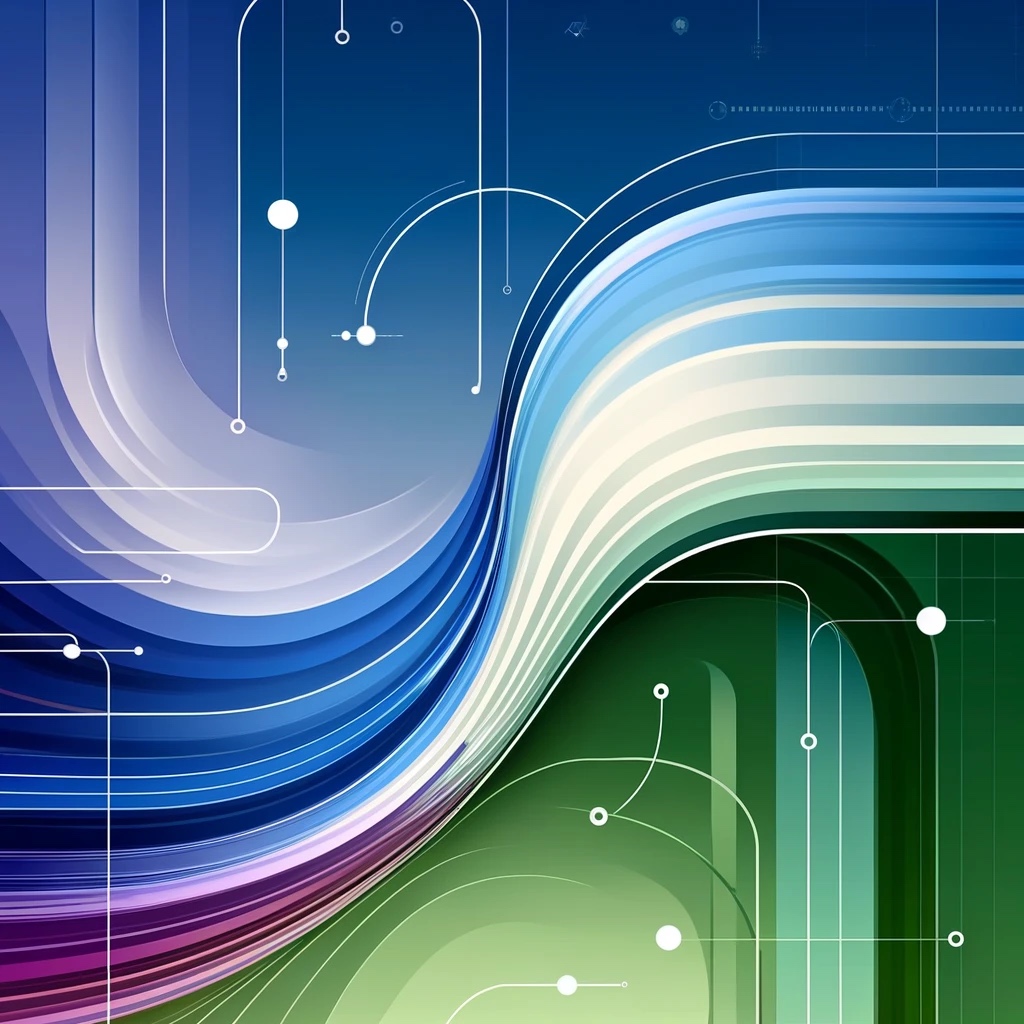
Gradients are a powerful tool in SwiftUI that allow you to create visually appealing backgrounds and effects. SwiftUI supports several types of gradients, including linear, radial, and angular gradients. This tutorial will guide you through the basics of using gradients in SwiftUI, with unique and interesting examples that are simple to understand for developers of all levels.
Introduction to Gradients in SwiftUI
SwiftUI provides built-in support for creating gradients using the LinearGradient
, RadialGradient
, and AngularGradient
views. These gradients can be used to fill shapes, backgrounds, and more.
Linear Gradient
A linear gradient creates a color transition along a straight line. You can define the start and end points, as well as the colors to transition between.
Example: Creating a Linear Gradient
import SwiftUI
struct LinearGradientView: View {
var body: some View {
Rectangle()
.fill(
LinearGradient(
gradient: Gradient(colors: [Color.red, Color.blue]),
startPoint: .topLeading,
endPoint: .bottomTrailing
)
)
.frame(width: 200, height: 200)
}
}
In this example, we create a Rectangle
filled with a linear gradient that transitions from red to blue, starting from the top leading corner to the bottom trailing corner.
Radial Gradient
A radial gradient creates a color transition radiating outward from a center point.
Example: Creating a Radial Gradient
import SwiftUI
struct RadialGradientView: View {
var body: some View {
Circle()
.fill(
RadialGradient(
gradient: Gradient(colors: [Color.yellow, Color.orange]),
center: .center,
startRadius: 20,
endRadius: 100
)
)
.frame(width: 200, height: 200)
}
}
In this example, we create a Circle
filled with a radial gradient that transitions from yellow at the center to orange at the edges.
Angular Gradient
An angular gradient creates a color transition around a circle, sweeping from one color to another.
Example: Creating an Angular Gradient
import SwiftUI
struct AngularGradientView: View {
var body: some View {
Circle()
.fill(
AngularGradient(
gradient: Gradient(colors: [Color.purple, Color.pink, Color.purple]),
center: .center
)
)
.frame(width: 200, height: 200)
}
}
In this example, we create a Circle
filled with an angular gradient that transitions from purple to pink and back to purple around the center.
Using EllipticalGradient with Shapes
Elliptical gradients can be applied to various shapes, not just ellipses. You can use them with rectangles, circles, and custom shapes to create unique visual effects.
Example: Applying Elliptical Gradient to a Rectangle
import SwiftUI
struct EllipticalGradientRectangleView: View {
var body: some View {
Rectangle()
.fill(
EllipticalGradient(
gradient: Gradient(colors: [.green, .yellow]),
center: .center,
startRadiusFraction: 0.2,
endRadiusFraction: 0.8
)
)
.frame(width: 300, height: 200)
}
}
Combining Gradients
You can combine different gradients to create more complex and interesting effects.
Example: Combining Linear and Radial Gradients
struct CombinedGradientView: View {
var body: some View {
ZStack {
Rectangle()
.fill(
LinearGradient(
gradient: Gradient(colors: [Color.blue.opacity(0.5), Color.green.opacity(0.5)]),
startPoint: .top,
endPoint: .bottom
)
)
.frame(width: 300, height: 300)
Circle()
.fill(
RadialGradient(
gradient: Gradient(colors: [Color.white.opacity(0.5), Color.clear]),
center: .center,
startRadius: 50,
endRadius: 150
)
)
.frame(width: 300, height: 300)
}
}
}
In this example, we create a Rectangle
with a linear gradient background and overlay a Circle
with a radial gradient to create a more complex visual effect.
Conclusion
Gradients are a versatile tool in SwiftUI that can enhance the visual appeal of your app. By understanding how to use LinearGradient
, RadialGradient
, and AngularGradient
, you can create stunning effects and backgrounds.