SwiftUI offers a variety of semantic colors that adapt to different contexts and system settings,…
Fonts in SwiftUI – System Fonts, Custom Fonts, Font Designs, Font Style
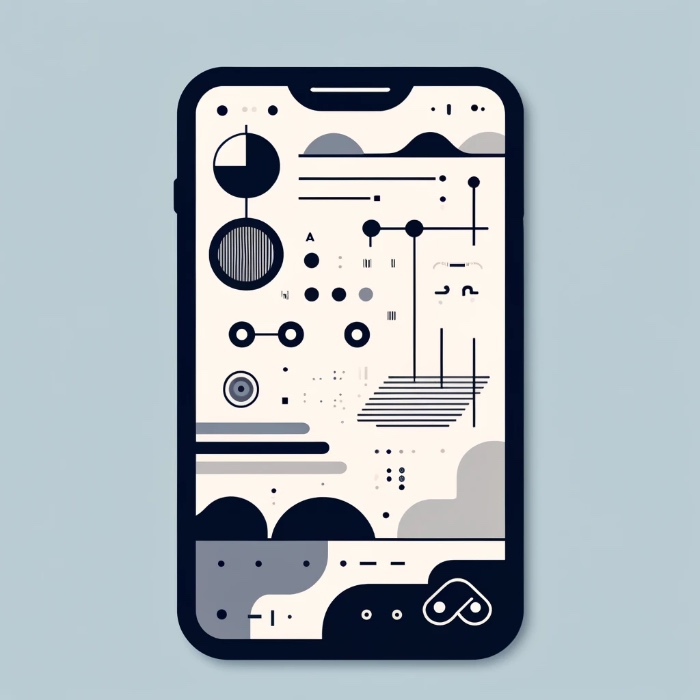
In SwiftUI, managing fonts effectively is essential for crafting visually appealing and functional user interfaces. Fonts contribute significantly to the readability and aesthetic of your app, making it important to understand how to utilize them correctly. This tutorial will guide you through the basics of using fonts in SwiftUI, including system fonts, custom fonts, and dynamic type adjustments for accessibility.
Using System Fonts
SwiftUI provides a robust set of system fonts that are optimized for different text sizes and weights. These fonts are designed to automatically adjust to the user’s accessibility settings, ensuring that your app remains accessible and easy to use for everyone.
Example: System Fonts in Use
struct ContentView: View {
var body: some View {
VStack(alignment: .leading, spacing: 10) {
Text("Welcome to SwiftUI!").font(.title)
Text("This text uses the default system font.")
Text("Here's a subtitle").font(.subheadline)
}
}
}
In this example, the Text
views are using different system font styles like .title
and .subheadline
, which are tailored to specific UI elements, ensuring that the text is both aesthetically pleasing and functionally effective.
SwiftUI offers a variety of predefined text styles that automatically adjust size and weight to match the user interface:
- .largeTitle: Commonly used for titles in navigation bars and headers.
- .title: Standard hierarchical heading.
- .title2: Secondary level of hierarchical headings.
- .title3: Third level of hierarchical headings.
- .headline: Used for headings, slightly bolder than Body.
- .subheadline: Typically used for subheadings, slightly smaller than Body.
- .body: Default style for body text in an app.
- .callout: Used for callouts, slightly larger than Body.
- .caption: For captions and secondary text, smaller than Body.
- .caption2: Alternative style for captions, slightly smaller than Caption.
- .footnote: Used in footnotes, the smallest text style.
Adding and Using Custom Fonts in SwiftUI
While system fonts are convenient, you might want to add a personal touch or match a specific design style by incorporating custom fonts into your SwiftUI app.
How to Add Custom Fonts:
- Add Font Files: Drag and drop your custom font files (
.ttf
or.otf
) into your Xcode project. - Update Info.plist: Register your fonts in the
Info.plist
underFonts provided by application
orUIAppFonts
. - Use the Font: Apply your custom font to text elements using the
.font()
modifier.
Step 2 Disclosure:
To use custom fonts in your SwiftUI app, it’s essential to properly register them in your project’s Info.plist
file. This step ensures that the fonts are bundled correctly with your application and are accessible to use within your SwiftUI views. Here’s how you can update your Info.plist
to register your custom fonts:
Steps to Register Fonts in Info.plist
:
- Open Your Project in Xcode:
- Navigate to the project navigator and select your project.
- Locate the
Info.plist
File:Info.plist
is usually found under the main app target. Click on it to view its contents.
- Add Fonts to
Info.plist
:- Click on the “+” icon to add a new entry if
Fonts provided by application
(orUIAppFonts
for the raw key) isn’t already present. - You will create a new array entry with this key.
- Click on the “+” icon to add a new entry if
- Specify Your Font Files:
- For each font file you want to use, add the font file name as a string item to the array. This includes the file extension. For example, if your font file is named
CustomFont-Regular.ttf
, you should add this exact string to the array.
- For each font file you want to use, add the font file name as a string item to the array. This includes the file extension. For example, if your font file is named
<key>UIAppFonts</key>
<array>
<string>CustomFont-Regular.ttf</string>
<string>CustomFont-Bold.ttf</string>
</array>
Example of Adding Fonts in Info.plist
:
Here’s what the entry might look like in your Info.plist
:
Example: Applying a Custom Font
struct CustomFontView: View {
var body: some View {
Text("Hello, custom font!")
.font(.custom("YourCustomFontName", size: 18))
}
}
This simple setup demonstrates how to implement a custom font within a Text
view. Be sure to replace "YourCustomFontName"
with the actual name of your font.
Dynamic Type and Accessibility
Dynamic Type is a powerful feature that adjusts the text size based on the user’s preferences, which can be particularly helpful for accessibility. SwiftUI makes it easy to integrate Dynamic Type in your app, ensuring your fonts scale correctly across different device settings.
Example: Dynamic Type with Custom Fonts
struct DynamicFontView: View {
var body: some View {
Text("Accessible font scaling!")
.font(.system(size: 16, weight: .medium, design: .rounded))
.scaledFont(name: "YourCustomFontName", size: 16)
}
}
In this example, the .scaledFont
modifier is used to apply Dynamic Type capabilities to a custom font, ensuring it respects user settings for text size.
Dynamic Type, which allows users to set their preferred text sizes, makes scaling especially important.
Using Font.custom(_:size:relativeTo:)
This method allows a custom font to scale relative to a predefined text style, ensuring that your app’s typography remains balanced and accessible. The relativeTo
parameter aligns the custom font’s size changes with the system’s text style changes, accommodating user preferences seamlessly.
Here’s a practical example to demonstrate how to effectively scale custom fonts with Dynamic Type:
VStack(alignment: .leading, spacing: 20) {
Group {
Text("Heading")
.font(.custom("AvenirNext-DemiBold", size: 24, relativeTo: .title))
Text("Subheading")
.font(.custom("AvenirNext-Regular", size: 18, relativeTo: .headline))
Text("Body Text")
.font(.custom("AvenirNext-Regular", size: 16, relativeTo: .body))
}
Group {
Text("Detailed Description")
.font(.custom("AvenirNext-Regular", size: 14, relativeTo: .footnote))
Text("Small Print")
.font(.custom("AvenirNext-Regular", size: 12, relativeTo: .caption))
}
}
.padding()
Exploring Font Weights in SwiftUI
SwiftUI provides a range of font weights that allow you to emphasize different parts of your UI by adjusting the thickness of the text. Here’s a list of the font weights available:
- UltraLight: Thinnest weight, less commonly used but effective for a delicate aesthetic.
- Thin: Slightly bolder than UltraLight, providing subtle emphasis without being too heavy.
- Light: Lighter than regular but heavier than Thin, suitable for secondary text that needs distinction.
- Regular: The standard font weight used in most text.
- Medium: Noticeably thicker than Regular, often used for highlighting information.
- Semibold: Stronger than Medium, great for drawing attention to specific text.
- Bold: Used to make text stand out prominently on your interface.
- Heavy: Heavier than Bold, used sparingly for maximum impact.
- Black: The boldest weight, used for very focused attention in titles or headings.
To illustrate the usage of font weights, let’s use Semibold
in an example within a SwiftUI view:
Text("Welcome to our app!")
.fontWeight(.semibold)
.font(.title)
Font Designs
SwiftUI allows you to select from different font designs, tailoring the feel of your text to match your app’s theme:
- Default: The standard system font design.
- Monospaced: Every character takes up the same horizontal space, good for code and numbers.
- Rounded: Features rounded edges, giving a soft, approachable look.
- Serif: Features small lines at the ends of strokes, traditional and formal.
Here’s how you can apply font designs:
Text("Rounded Font Style")
.font(.system(.title, design: .rounded))
Text("Monospace Font Style")
.font(.system(.title, design: .monospaced))
Text("Serif Font Style")
.font(.system(.title, design: .serif))
Text("Default Font Style")
.font(.system(.title, design: .default))
Conclusion
Fonts in SwiftUI not only enhance the visual appeal of your app but also improve its usability and accessibility. By understanding how to use both system and custom fonts effectively, and integrating Dynamic Type, you can ensure that your app offers a great user experience for all users. Experiment with different fonts and settings to discover what works best for your specific app design needs.