SwiftUI offers a variety of semantic colors that adapt to different contexts and system settings,…
Factory Pattern in SwiftUI
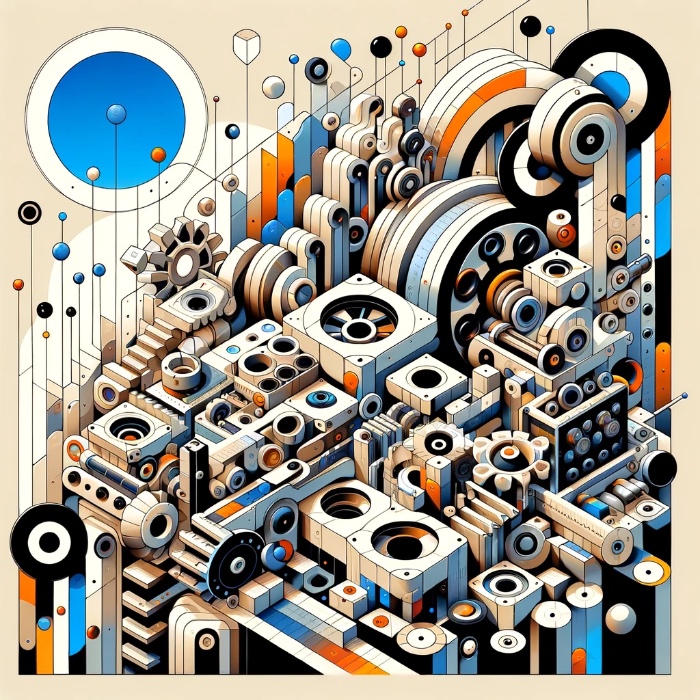
In the SwiftUI ecosystem, crafting intuitive and interactive user interfaces often requires a dynamic approach to component creation and management. The Factory Pattern, a cornerstone in the realm of software design patterns, offers a streamlined solution for this challenge, akin to a well-oiled assembly line in a manufacturing plant, meticulously producing various products to meet specific demands.
The Factory Pattern: A Catalyst for Modular SwiftUI Development
Picture yourself navigating the vast corridors of an advanced manufacturing facility, where each section is dedicated to assembling a distinct model of vehicle, tailored to precise specifications. This analogy parallels the Factory Pattern’s role in programming, where it abstracts the instantiation process, enabling the creation of objects without necessitating knowledge of the underlying class that produces them.
Implementing the Factory Pattern: A SwiftUI Scenario
Let’s delve into an illustrative example of employing the Factory Pattern within a SwiftUI application, aimed at producing a versatile and engaging animal sound generator.
Defining the Blueprint: The AnimalSound
Protocol
Initially, we outline a protocol to serve as a blueprint for our sound-producing entities, ensuring uniformity across all implementations:
protocol AnimalSound {
func makeSound() -> String
}
Crafting the Implementations: CatSound
and DogSound
Following the protocol, we instantiate concrete classes that adhere to the specified blueprint, each encapsulating the logic to produce a unique animal sound:
struct CatSound: AnimalSound {
func makeSound() -> String { "Meow!" }
}
struct DogSound: AnimalSound {
func makeSound() -> String { "Woof!" }
}
The Factory at Work: AnimalSoundFactory
The Factory Pattern comes into play, dictating the creation logic and deciding the specific product to generate based on the provided identifier:
enum AnimalType {
case cat, dog
}
class AnimalSoundFactory {
static func createAnimalSound(for type: AnimalType) -> AnimalSound {
switch type {
case .cat:
return CatSound()
case .dog:
return DogSound()
}
}
}
Bringing It All Together in SwiftUI
The Factory’s versatility shines in a SwiftUI view, allowing users to select their preferred animal and hear the corresponding sound:
struct ContentView: View {
@State private var selectedAnimal: AnimalType = .cat
@State private var animalSound: String = ""
var body: some View {
VStack {
Picker("Select an animal", selection: $selectedAnimal) {
Text("Cat").tag(AnimalType.cat)
Text("Dog").tag(AnimalType.dog)
}
.pickerStyle(SegmentedPickerStyle())
.padding()
Button("Make Sound") {
let animal = AnimalSoundFactory.createAnimalSound(for: selectedAnimal)
animalSound = animal.makeSound()
}
Text(animalSound)
.font(.largeTitle)
.padding()
}
}
}
Learn more: App Design Patterns in SwiftUI