SwiftUI offers a variety of semantic colors that adapt to different contexts and system settings,…
Facade Pattern in SwiftUI
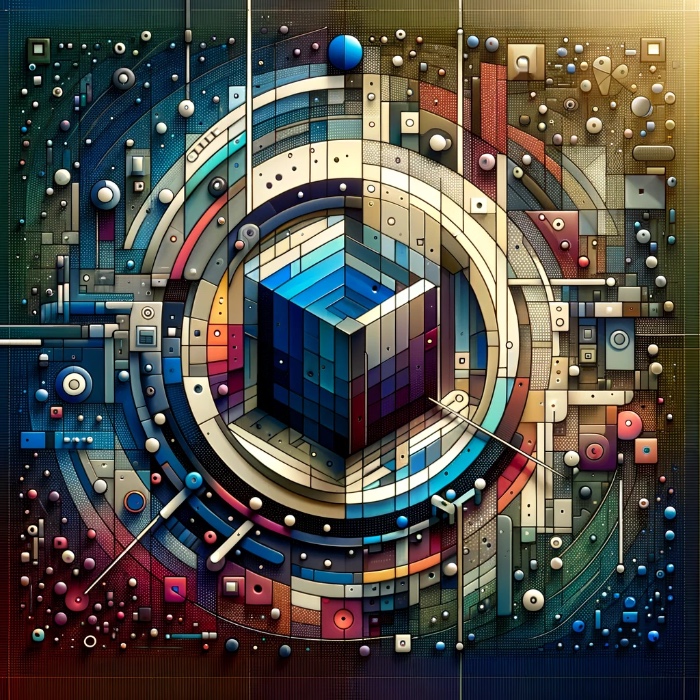
Imagine trying to manage a sophisticated home theater setup with a different remote for the TV, sound system, and streaming device. The Facade Pattern is akin to replacing this cumbersome process with a single, streamlined remote offering one-button solutions. In programming, especially within the SwiftUI framework, it harmonizes interactions with complex systems, allowing for ease of use without diluting underlying functionalities.
SwiftUI Weather Display: A Facade Pattern Implementation
To demonstrate the Facade Pattern’s prowess, let’s walk through a SwiftUI example that fetches and displays weather information. This scenario involves coordinating multiple services—temperature, humidity, and weather conditions—into a cohesive and user-friendly interface.
Step 1: Laying the Groundwork with Subsystems
Our first task is to define the subsystems responsible for delivering distinct weather metrics:
class TemperatureService {
func getTemperature() -> String { "72°F" }
}
class HumidityService {
func getHumidity() -> String { "65%" }
}
class ConditionService {
func getCondition() -> String { "Sunny" }
}
Step 2: Crafting the Facade
With our subsystems in place, we then construct a Facade, WeatherFacade
, to abstract the complexities of individual service interactions:
class WeatherFacade {
private let temperatureService = TemperatureService()
private let humidityService = HumidityService()
private let conditionService = ConditionService()
func getWeatherInfo() -> String {
let temp = temperatureService.getTemperature()
let humidity = humidityService.getHumidity()
let condition = conditionService.getCondition()
return "It's \(temp) with \(humidity) humidity and \(condition) outside."
}
}
Step 3: Integrating the Facade within SwiftUI
Finally, we employ the Facade in a SwiftUI view, enabling a seamless display of integrated weather information:
import SwiftUI
struct ContentView: View {
private let weatherFacade = WeatherFacade()
var body: some View {
VStack {
Text("Current Weather:")
.font(.headline)
Text(weatherFacade.getWeatherInfo())
.font(.title)
}
.padding()
}
}
Learn more:Â App Design Patterns in SwiftUI