SwiftUI offers a variety of semantic colors that adapt to different contexts and system settings,…
ContentUnavailableView in SwiftUI: Enhance UX During Data Downtime
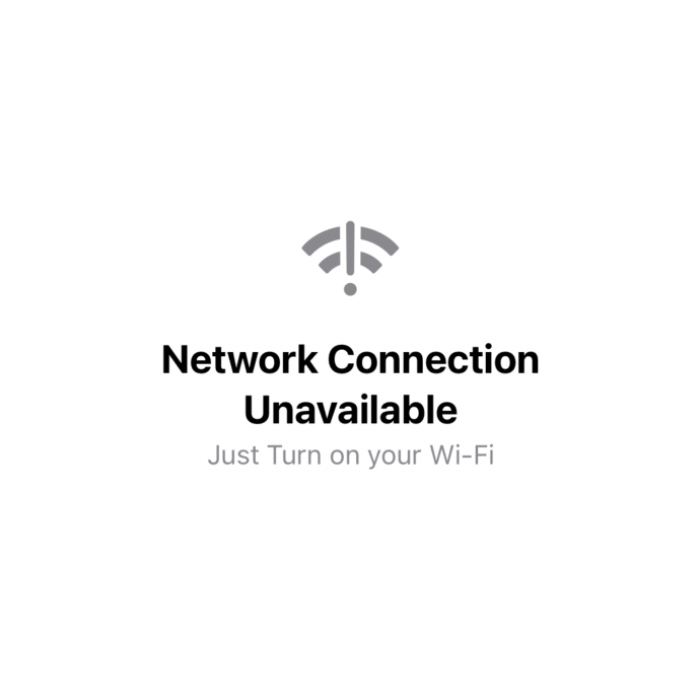
In app development, handling situations where data is temporarily unavailable is crucial for maintaining a good user experience. SwiftUI’s ContentUnavailableView
provides a streamlined way to inform users when content cannot be displayed, such as during network failures or when search results are empty. This tutorial will guide you through creating an engaging ContentUnavailableView
that keeps users informed and engaged, even when the data they need isn’t available.
When to Use ContentUnavailableView
ContentUnavailableView
should be utilized whenever your app cannot display its usual content. This might occur under various circumstances such as:
- A network connection error.
- An empty list that usually displays data.
- A search operation that returns no results.
Implementing ContentUnavailableView
in these situations helps maintain a smooth and informative user experience, preventing user frustration and confusion.
Built-in Unavailable Views
SwiftUI provides a default ContentUnavailableView
specifically for search scenarios, which is straightforward to implement.
struct ContentUnavailableViewBasic: View {
var body: some View {
ContentUnavailableView.search
}
}
struct ContentUnavailableViewBasicWithText: View {
var body: some View {
ContentUnavailableView.search(text: "Some Text")
}
}
Custom Unavailable Views
To create a custom ContentUnavailableView
for situations other than searches, you can utilize SwiftUI’s flexibility to define specific labels, images, and descriptions. For example, to display a view for a network error:
ContentUnavailableView(
"Network Connection Unavailable",
systemImage: "wifi.exclamationmark",
description: Text("Just Turn on your Wi-Fi")
)
Unavailable Views with Actions
Adding actions to ContentUnavailableView
allows you to direct users towards potential solutions or alternative actions. Here’s how you can offer a proactive approach in your unavailable view:
Let’s create a custom ContentUnavailableView
for a hypothetical gardening app where users can track and manage their plants. In this example, we’ll design an unavailable view for when there are no plants in a user’s garden. The view will encourage users to explore available plants and add them to their garden.
Custom Unavailable View for a Gardening App
This SwiftUI example is tailored for situations where the plant list is empty, perhaps because the user is new to the app or hasn’t added any plants yet.
struct GardenView_CustomContentUnavailable: View {
@State private var plants: [String] = []
@State private var isLoading = true
var body: some View {
NavigationView {
List(plants, id: \.self) { plant in
Text(plant)
}
.navigationTitle("Your Garden")
.overlay(emptyGardenOverlay)
.onAppear {
loadPlants()
}
}
}
private var emptyGardenOverlay: some View {
Group {
if plants.isEmpty && !isLoading {
ContentUnavailableView {
Label("No Plants Found", systemImage: "leaf.arrow.circlepath")
} description: {
Text("Your garden is empty. Start by exploring our plant catalog to find your next green friend.")
} actions: {
Button("Explore Plants") {
// Action to navigate to the plant catalog section
}
.buttonStyle(.borderedProminent)
}
}
}
}
private func loadPlants() {
// Simulate loading plants
DispatchQueue.main.asyncAfter(deadline: .now() + 2) {
self.plants = [] // Simulate no results
self.isLoading = false
}
}
}
Explanation:
- Navigation View & List: This structure holds a list that attempts to display plants within the user’s garden.
- Loading Simulation: When
GardenView
appears,loadPlants()
mimics fetching plant data. After a delay, it sets theplants
array as empty and updatesisLoading
to false. - Overlay: When the plant list is empty and not loading,
ContentUnavailableView
overlays the list with a custom message and actions. - Custom Unavailable View: The view contains a label with a system image and a description that encourages users to add plants to their empty garden. Additionally, it includes an actionable button labeled “Explore Plants,” which could be hooked up to navigate to a plant catalog.
This example effectively demonstrates how to use ContentUnavailableView
to handle empty states in an app, providing users with guidance and actions to improve their experience.
Conclusion
ContentUnavailableView
in SwiftUI provides a significant opportunity to enhance user experience during data downtime or when content is otherwise unavailable. By implementing both built-in and custom views, and incorporating actionable solutions, developers can maintain user engagement and minimize potential frustration. Experiment with different configurations to tailor the ContentUnavailableView
to fit seamlessly within your app, ensuring it aligns with the overall design and user expectations.