SwiftUI offers a variety of semantic colors that adapt to different contexts and system settings,…
Clipboard – Copy and Paste in SwiftUI
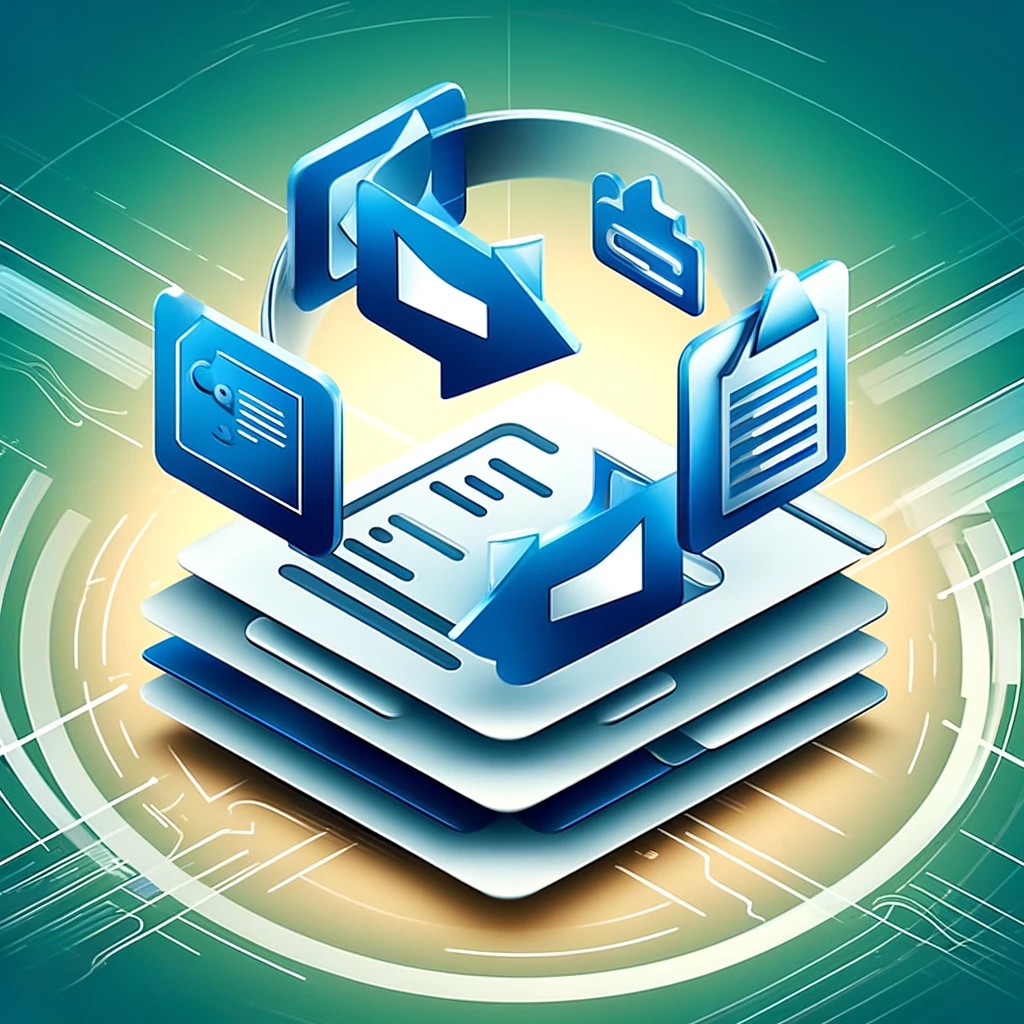
SwiftUI provides simple and powerful APIs to interact with the system clipboard. You can easily copy text to the clipboard and retrieve text from it.
Basic Usage: Copying Text to Clipboard
Let’s start with a basic example where we copy a text string to the clipboard when a button is pressed.
Example: Copying Text to Clipboard
import SwiftUI
struct CopyTextView: View {
@State private var textToCopy = "Hello, SwiftUI!"
@State private var message = "Tap the button to copy text"
var body: some View {
VStack(spacing: 20) {
Text(message)
.padding()
Text(textToCopy)
.padding()
.background(Color.gray.opacity(0.2))
.cornerRadius(10)
Button(action: {
UIPasteboard.general.string = textToCopy
message = "Text copied to clipboard!"
}) {
Text("Copy Text")
.padding()
.background(Color.blue)
.foregroundColor(.white)
.cornerRadius(10)
}
}
.padding()
}
}
In this example:
- We define a
@State
variabletextToCopy
that holds the text we want to copy. - Another
@State
variablemessage
is used to display the status message. - When the button is pressed, the text is copied to the clipboard using
UIPasteboard.general.string
.
Combining Copy and Paste Functionality
Let’s combine the copy and paste functionality into a single view.
Example: Copy and Paste Text
import SwiftUI
struct CopyPasteTextView: View {
@State private var textToCopy = "Hello, SwiftUI!"
@State private var pastedText = "Tap 'Paste' to see clipboard text"
var body: some View {
VStack(spacing: 20) {
Text("Text to Copy:")
Text(textToCopy)
.padding()
.background(Color.gray.opacity(0.2))
.cornerRadius(10)
Button(action: {
UIPasteboard.general.string = textToCopy
}) {
Text("Copy Text")
.padding()
.background(Color.blue)
.foregroundColor(.white)
.cornerRadius(10)
}
Divider()
Text("Pasted Text:")
Text(pastedText)
.padding()
.background(Color.gray.opacity(0.2))
.cornerRadius(10)
Button(action: {
if let text = UIPasteboard.general.string {
pastedText = text
} else {
pastedText = "No text found in clipboard"
}
}) {
Text("Paste Text")
.padding()
.background(Color.green)
.foregroundColor(.white)
.cornerRadius(10)
}
}
.padding()
}
}
In this example:
- We define two
@State
variablestextToCopy
andpastedText
. - The “Copy Text” button copies
textToCopy
to the clipboard. - The “Paste Text” button retrieves and displays text from the clipboard in the
pastedText
variable.
Conclusion
Using the clipboard in SwiftUI is straightforward and enhances the interactivity of your app. By understanding how to copy and paste text, you can create more user-friendly interfaces.