SwiftUI offers a variety of semantic colors that adapt to different contexts and system settings,…
Builder Pattern in SwiftUI
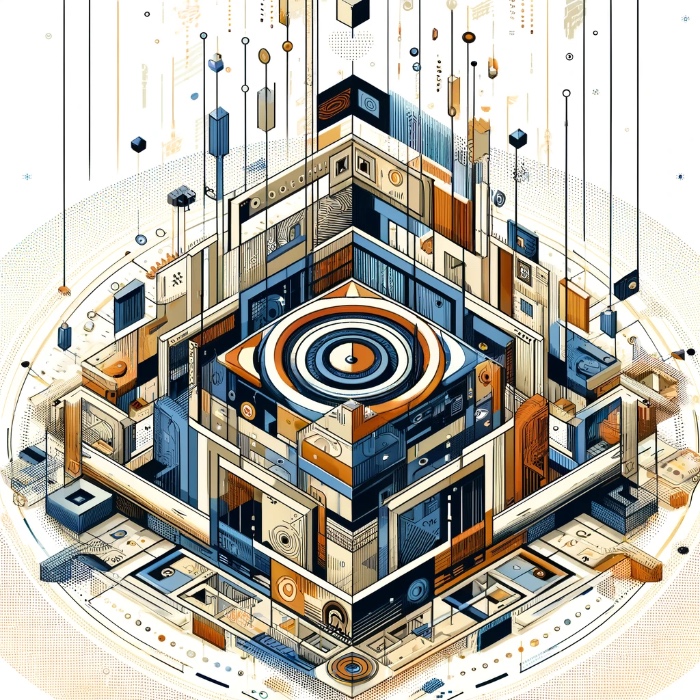
Creating complex and customizable user interfaces in SwiftUI can sometimes resemble the intricacies of crafting a gourmet meal. Each element or component within your app’s UI can be seen as an ingredient that contributes to the overall user experience. The Builder Pattern emerges as a culinary wizard in this scenario, streamlining the process of UI construction, ensuring every ingredient is perfectly placed according to your app’s recipe for success.
The Essence of the Builder Pattern in SwiftUI Development
Imagine the process of assembling a deluxe sandwich, where each layer, from the choice of bread to the variety of fillings, is selected to match your taste preferences perfectly. The Builder Pattern in SwiftUI operates on a similar principle, allowing developers to construct UI components with precision, specifying attributes step-by-step to achieve the desired outcome without overwhelming the initial setup call with parameters.
Crafting Custom UI Elements with the Builder Pattern
To bring this concept to life, let’s delve into a practical application within SwiftUI, illustrating how the Builder Pattern can be harnessed to create customizable text elements.
The CustomTextBuilder Blueprint
Our journey begins with defining a CustomTextBuilder
, a struct designed to construct text views tailored to specific stylistic preferences:
struct CustomTextBuilder {
private var text: String
private var font: Font = .body
private var textColor: Color = .black
private var alignment: TextAlignment = .leading
init(_ text: String) {
self.text = text
}
func font(_ font: Font) -> CustomTextBuilder {
var builder = self
builder.font = font
return builder
}
func textColor(_ color: Color) -> CustomTextBuilder {
var builder = self
builder.textColor = color
return builder
}
func multilineTextAlignment(_ alignment: TextAlignment) -> CustomTextBuilder {
var builder = self
builder.alignment = alignment
return builder
}
func build() -> some View {
Text(text)
.font(font)
.foregroundColor(textColor)
.multilineTextAlignment(alignment)
}
}
This builder equips developers with a fluent interface to configure text elements — from font size and color to alignment — in a clear and concise manner.
Implementing in SwiftUI Views
Utilizing the CustomTextBuilder
within a SwiftUI view showcases its potential to enhance both code readability and UI customization:
struct ContentView: View {
var body: some View {
VStack {
CustomTextBuilder("Welcome to SwiftUI Patterns!")
.font(.title)
.textColor(.blue)
.multilineTextAlignment(.center)
.build()
CustomTextBuilder("Embrace the power of the Builder Pattern.")
.font(.headline)
.textColor(.green)
.multilineTextAlignment(.leading)
.build()
}
}
}
Learn more: App Design Patterns in SwiftUI