SwiftUI offers a variety of semantic colors that adapt to different contexts and system settings,…
@AppStorage and @SceneStorage in SwiftUI
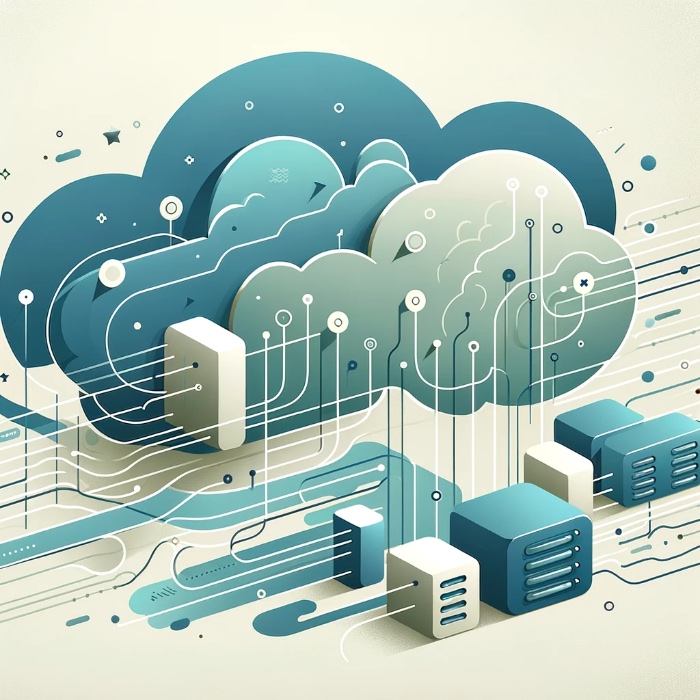
In SwiftUI, managing the state of your application through restarts and multitasking environments is crucial for creating a seamless user experience. SwiftUI provides two powerful tools, @AppStorage
and @SceneStorage
, designed to help developers handle state preservation and restoration efficiently. This tutorial will explore how to use these tools to save and retrieve user interface state across app launches and during active sessions.
Using @AppStorage for Persistent State Management
@AppStorage
reads and writes values to UserDefaults
, automatically updating your view when values change. It is ideal for storing simple data types and user preferences that need to persist between app launches.
Example: Storing User Preferences with @AppStorage
import SwiftUI
struct SettingsView: View {
@AppStorage("darkModeEnabled") private var darkModeEnabled: Bool = false
var body: some View {
Toggle("Enable Dark Mode", isOn: $darkModeEnabled)
.padding()
}
}
What’s Happening:
- The
Toggle
control is bound todarkModeEnabled
, stored inUserDefaults
under the key"darkModeEnabled"
. - Changes to the toggle will automatically save to
UserDefaults
and the view will update accordingly to reflect the user’s preference.
Using @SceneStorage for Scene-Specific State Restoration
@SceneStorage
is used to store data specific to a scene, which is useful for apps that support multiple windows or need to manage state in complex multi-scene setups like on iPadOS.
Example: Preserving Text Editor State with @SceneStorage
import SwiftUI
struct EditorView: View {
@SceneStorage("editorContent") private var editorContent: String = ""
var body: some View {
NavigationStack {
TextEditor(text: $editorContent)
.padding()
}
}
}
What’s Happening:
TextEditor
is bound toeditorContent
, which is stored and restored automatically for each scene instance using@SceneStorage
.- If the user edits text in one window, closes the app, and reopens it, the text they were editing will be preserved and restored.
Practical Considerations
- Data Security: Neither
@AppStorage
nor@SceneStorage
should be used for storing sensitive data as they do not provide secure storage. - Data Volume: It is recommended to only store minimal data necessary for restoring state. Large data sets should be managed differently, possibly using databases or more secure storage solutions.
Conclusion
Using @AppStorage
and @SceneStorage
in SwiftUI enables developers to manage app state effectively, providing continuity for users across app launches and maintaining individual scene states in multi-window environments. By integrating these tools, you can enhance the robustness and user-friendliness of your SwiftUI applications. Experiment with these property wrappers to find the best ways to keep your app’s user interface responsive and intuitive.