SwiftUI offers a variety of semantic colors that adapt to different contexts and system settings,…
App Lifecycle in SwiftUI: WindowGroup, Scenes and Scene Phases
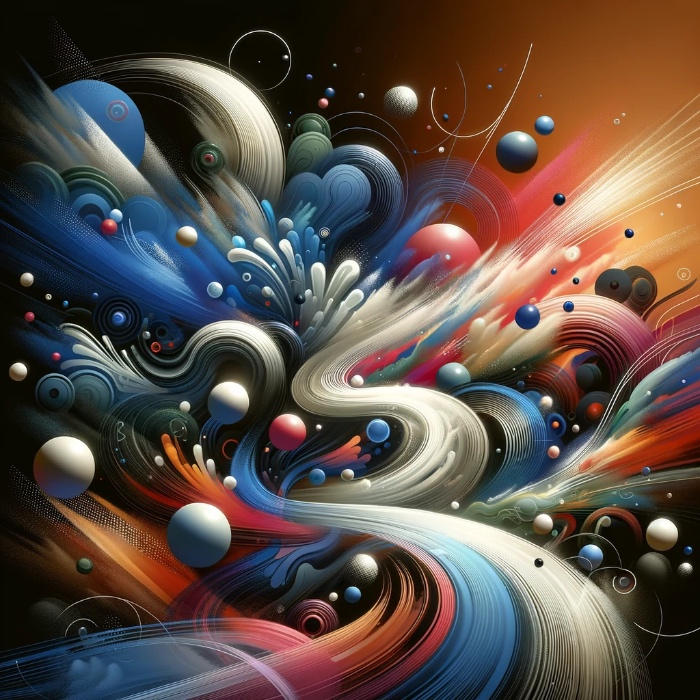
SwiftUI introduces a modern and streamlined way to manage the lifecycle of iOS applications.
The SwiftUI App Lifecycle Overview
In SwiftUI, the entry point of your application is marked by the @main
attribute, which identifies the App struct. This struct conforms to the App protocol, encapsulating the configuration and behavior of your application. Within the App struct, you define your app’s body, typically consisting of one or more scenes.
Scenes in SwiftUI
A scene represents a single instance of your app’s user interface. SwiftUI apps can contain multiple scenes, though most applications will primarily use a single window or view. The most common scene type in iOS development is WindowGroup
, which represents a group of windows that share the same content.
@main
struct MyApp: App {
var body: some Scene {
WindowGroup {
ContentView()
}
}
}
What is a WindowGroup?
In SwiftUI, a WindowGroup
is used as a container for the view hierarchy your app presents. When you declare a view hierarchy within a WindowGroup
, you’re essentially providing SwiftUI with a template for creating windows in your app. This concept is crucial in multiplatform apps, particularly those that run on macOS and iPadOS, where multiple windows of the same app can be open simultaneously.
The WindowGroup
acts as a flexible and dynamic container that automatically adjusts to the environment it’s running in. For example, in a macOS app, the WindowGroup
can lead to the creation of multiple app windows, each hosting the declared view hierarchy. In iOS, while the concept of multiple windows is not as prevalent due to the single-window nature of most iOS apps, WindowGroup
ensures your app’s main content is appropriately managed and displayed.
WindowGroup Provides several benefits:
- Template for Window Creation: As mentioned, the view hierarchy declared in a
WindowGroup
serves as a template. This is particularly useful in environments supporting multiple windows, ensuring consistency across different instances of your app’s UI. - Automatic Management: SwiftUI takes care of managing the lifecycle of windows created from a
WindowGroup
. This includes handling user interactions that create, close, or switch between windows in environments that support such actions. - Adaptive UI: The content within a
WindowGroup
automatically adapts to the platform it’s running on. This means the same SwiftUI code can result in a multi-window application on macOS or iPadOS, while on iOS, it adapts to a single-window layout without additional code.
Understanding Scene Phases in SwiftUI
SwiftUI introduces the concept of scene phases, allowing developers to respond to changes in a scene’s lifecycle. The ScenePhase
enum includes several cases such as active
, inactive
, and background
, corresponding to the various states your app can be in. By monitoring these phases, you can execute specific code when your app transitions between them.
To respond to scene phase changes, you use the .onChange(of:)
modifier on your scene, often in the WindowGroup
. This requires injecting the environment value scenePhase
into your scene:
@main
struct MyApp: App {
@Environment(\.scenePhase) var scenePhase
var body: some Scene {
WindowGroup {
ContentView()
}
.onChange(of: scenePhase) {
switch scenePhase {
case .active:
print("App is active")
case .inactive: //
print("App is inactive")
case .background:
print("App is in the background")
@unknown default:
print("A new, unknown scene phase")
}
}
}
}
Practical Uses of Scene Phases
Understanding and utilizing scene phases are crucial for managing tasks related to your app’s lifecycle effectively. For example:
- .active: Triggered when the app moves to the foreground and becomes interactive. Use this phase to start or resume tasks that should run while the app is visible, such as animations or refreshing data, track app launches, local notifications.
- .inactive: Occurs when the app is still in the foreground but not receiving events. This phase indicates that your app is running but not receiving events. It’s an opportunity to pause animations or reduce workloads.
- .background: The app is not visible to the user and should limit its activities. When your app moves to the background, it’s essential to free up resources, save user data, and prepare for termination if necessary.
Conclusion
The introduction of scenes and scene phases in SwiftUI represents a significant shift from the imperative approach of UIKit to a more declarative configuration of your app’s lifecycle. By understanding how to effectively use these tools, you can create responsive and efficient SwiftUI applications that gracefully handle lifecycle events. Whether you’re managing data persistence, adjusting UI based on app state, or conserving resources, leveraging scenes and scene phases allows for a clean and organized approach to app lifecycle management.