SwiftUI offers a variety of semantic colors that adapt to different contexts and system settings,…
Adapter Pattern in SwiftUI
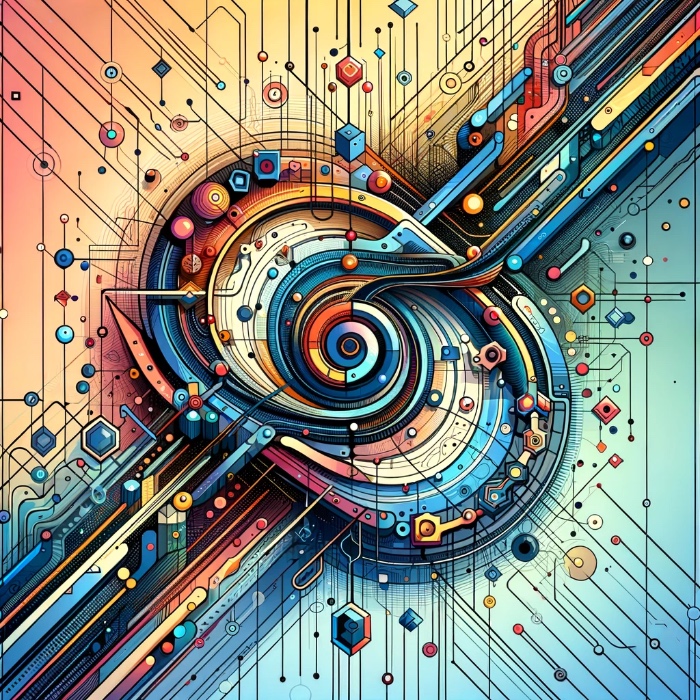
Imagine landing in a foreign country, your phone’s battery dwindling, only to discover the charger plug doesn’t match the local sockets. Just as a travel adapter saves the day by ensuring compatibility, the Adapter Pattern in programming enables disparate systems to work together harmoniously.
Transforming Data with the Adapter Pattern: A SwiftUI Temperature Conversion
Consider the task of integrating a Fahrenheit-based temperature reading into a SwiftUI app designed to display Celsius. The Adapter Pattern provides the perfect framework to reconcile these differences, facilitating a seamless data transformation.
Step 1: Establishing the Foundation with Legacy Systems
Our journey begins with a legacy temperature sensor, deeply entrenched in the Fahrenheit scale:
// Legacy system
class FahrenheitTemperatureSensor {
func getTemperatureFahrenheit() -> Double {
return 72.0 // Sample temperature in Fahrenheit
}
}
Step 2: Crafting the Adapter for Celsius Conversion
Next, we introduce the Adapter, a pivotal component that translates Fahrenheit readings into the Celsius scale:
// Adapter
class CelsiusTemperatureAdapter {
private var fahrenheitSensor: FahrenheitTemperatureSensor
init(fahrenheitSensor: FahrenheitTemperatureSensor) {
self.fahrenheitSensor = fahrenheitSensor
}
func getTemperatureCelsius() -> Double {
return (fahrenheitSensor.getTemperatureFahrenheit() - 32) * 5 / 9
}
}
Step 3: Integrating the Adapter within SwiftUI
With the Adapter in hand, we can now effortlessly incorporate Celsius temperature readings into our SwiftUI view:
import SwiftUI
struct ContentView: View {
private let sensor = FahrenheitTemperatureSensor()
private var adapter: CelsiusTemperatureAdapter
init() {
adapter = CelsiusTemperatureAdapter(fahrenheitSensor: sensor)
}
var body: some View {
VStack {
Text("Temperature")
.font(.headline)
Text("\(adapter.getTemperatureCelsius(), specifier: "%.1f") °C")
.font(.title)
}
}
}
This approach not only showcases the Adapter Pattern’s ability to facilitate interoperability within SwiftUI apps but also underscores its role in enhancing app functionality without the need for extensive rework or overhaul of existing systems.
Learn more:Â App Design Patterns in SwiftUI